Python PyQt button click example
Write a Python program that creates a button and when clicked it prints a message. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QPushButton Class: The QPushButton widget provides a command button.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout
from PyQt5.QtCore import pyqtSignal
class CustomWidget(QWidget):
# Define a custom signal
button_clicked = pyqtSignal()
def __init__(self):
super().__init__()
layout = QVBoxLayout()
# Create a custom button
self.custom_button = QPushButton("Custom Button", self)
layout.addWidget(self.custom_button)
# Connect the button's clicked signal to the custom signal
self.custom_button.clicked.connect(self.button_clicked)
self.setLayout(layout)
def custom_slot():
print("The custom button is clicked.")
def main():
app = QApplication(sys.argv)
window = CustomWidget()
window.setWindowTitle("Custom Widget Example")
window.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Connect the custom signal to the custom slot
window.button_clicked.connect(custom_slot)
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QWidget" class named "CustomWidget" that inherits from "QWidget". Inside this class, we define a custom signal called "button_clicked".
- Design a custom button called self.custom_button and connect its clicked signal to the custom ‘button_clicked’ signal.
- Define a custom slot function called "custom_slot()" that reacts to button presses. In this case, it prints a message when the button is clicked.
- In the main function, we create the PyQt application, instantiate the "CustomWidget" class, set the window properties, and display the main window.
Output:
The custom button is clicked. The custom button is clicked. The custom button is clicked.![]()
Flowchart:
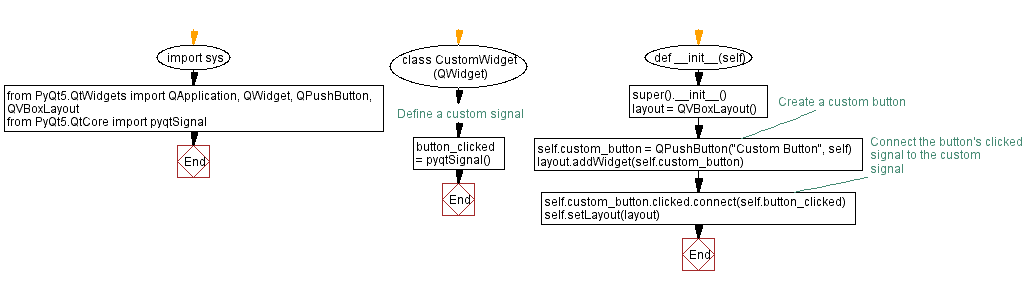
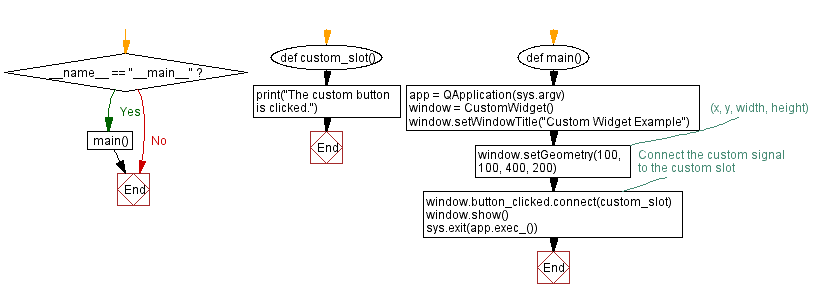
Python Code Editor:
Previous: Python PyQt comboBox example.
Next: Python PyQt custom signal example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics