Python PyQt custom signal example
Write a Python program that creates a button and when clicked sends a message with a specific value. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QPushButton Class: The QPushButton widget provides a command button.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton
from PyQt5.QtCore import pyqtSignal, QObject
class MySignalEmitter(QObject):
# Define a custom signal with a value
custom_signal = pyqtSignal(str)
class MyCustomWidget(QWidget):
def __init__(self):
super().__init__()
# Create an instance of the signal emitter
self.signal_emitter = MySignalEmitter()
# Create a button
button = QPushButton("Click Me", self)
button.clicked.connect(self.on_button_click)
def on_button_click(self):
# Emit the custom signal with a value
self.signal_emitter.custom_signal.emit("Exercises from w3resource!")
def custom_slot(value):
print("Python PyQt:", value)
def main():
app = QApplication(sys.argv)
window = MyCustomWidget()
window.setWindowTitle("Custom Signal Example")
window.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Connect the custom signal to the custom slot
window.signal_emitter.custom_signal.connect(custom_slot)
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom signal emitter class "MySignalEmitter", which defines a custom signal named 'custom_signal'. This signal can send a string value when emitted.
- Create a custom widget class "MyCustomWidget", which contains a button. When the button is clicked, it emits the custom_signal with the message "Exercises from w3resource!"
- Define a custom slot function "custom_slot()" that receives and prints the value sent by the signal.
- In the main() function, we create the PyQt application, instantiate the "MyCustomWidget" class, set the window properties, and connect the custom signal to the custom slot.
Output:
Python PyQt: Exercises from w3resource!![]()
Flowchart:
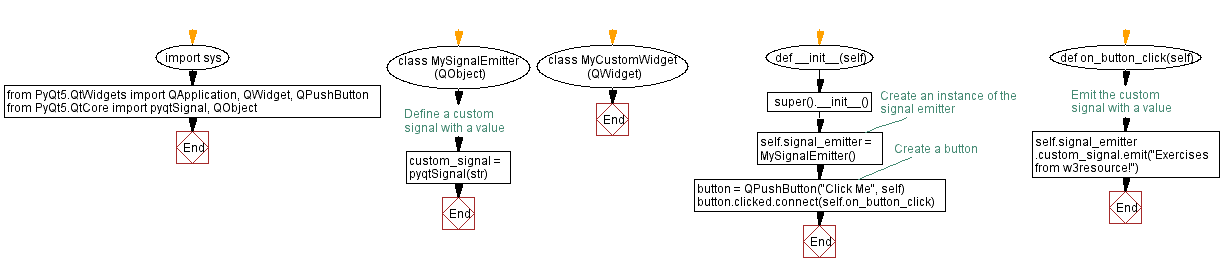
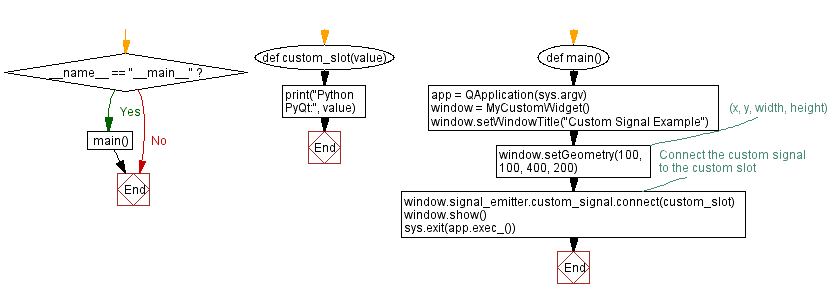
Go to:
Previous: Python PyQt button click example.
Next: Python PyQt character counter notepad.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.