Python PyQt simple calculator
Write a Python program that creates a simple calculator application and displays buttons for numbers and math operations. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QPushButton Class: The QPushButton widget provides a command button.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QGridLayout Class: The QGridLayout class lays out widgets in a grid.
QLabel Class: The QLabel widget provides a text or image display.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout, QGridLayout, QLabel
class CalculatorApp(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Simple Calculator")
self.setGeometry(100, 100, 300, 400) # (x, y, width, height)
layout = QVBoxLayout()
# Create a label to display the calculator input and result
self.display_label = QLabel("0", self)
layout.addWidget(self.display_label)
# Create a layout for the buttons
button_layout = QGridLayout()
# Create buttons for numbers 0-9
for i in range(10):
button = QPushButton(str(i), self)
button.clicked.connect(lambda checked, num=i: self.on_digit_clicked(num))
if i == 0:
button_layout.addWidget(button, 3, 1)
else:
button_layout.addWidget(button, (9 - i) // 3, (i - 1) % 3)
# Create buttons for operators (+, -, *, /)
operators = ["+", "-", "*", "/"]
for operator in operators:
button = QPushButton(operator, self)
button.clicked.connect(lambda checked, op=operator: self.on_operator_clicked(op))
button_layout.addWidget(button, operators.index(operator), 3)
# Create a button for equals (=)
equals_button = QPushButton("=", self)
equals_button.clicked.connect(self.calculate_result)
button_layout.addWidget(equals_button, 3, 2)
# Create a clear button (C)
clear_button = QPushButton("C", self)
clear_button.clicked.connect(self.clear_input)
button_layout.addWidget(clear_button, 3, 0)
layout.addLayout(button_layout)
self.setLayout(layout)
self.current_input = ""
def on_digit_clicked(self, digit):
self.current_input += str(digit)
self.display_label.setText(self.current_input)
def on_operator_clicked(self, operator):
if self.current_input and self.current_input[-1] not in ["+", "-", "*", "/"]:
self.current_input += operator
self.display_label.setText(self.current_input)
def calculate_result(self):
try:
result = eval(self.current_input)
self.display_label.setText(str(result))
self.current_input = str(result)
except Exception:
self.display_label.setText("Error")
self.current_input = ""
def clear_input(self):
self.current_input = ""
self.display_label.setText("0")
def main():
app = QApplication(sys.argv)
window = CalculatorApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QWidget" class named "CalculatorApp" that builds a simple calculator application.
- Design a layout with buttons for numbers (0-9), operators (+, -, *, /), an equals (=) button, and a clear (C) button.
- When we click the number buttons, operators, equals, or clear buttons, it updates the input and displays the result.
- We use a "QLabel" (self.display_label) to display input and results.
- The "on_digit_clicked()", "on_operator_clicked()", "calculate_result()", and "clear_input()" methods handle button clicks and perform calculations.
- In the main function, we create the PyQt application, instantiate the CalculatorApp class, and show the main window.
Output:
Flowchart:
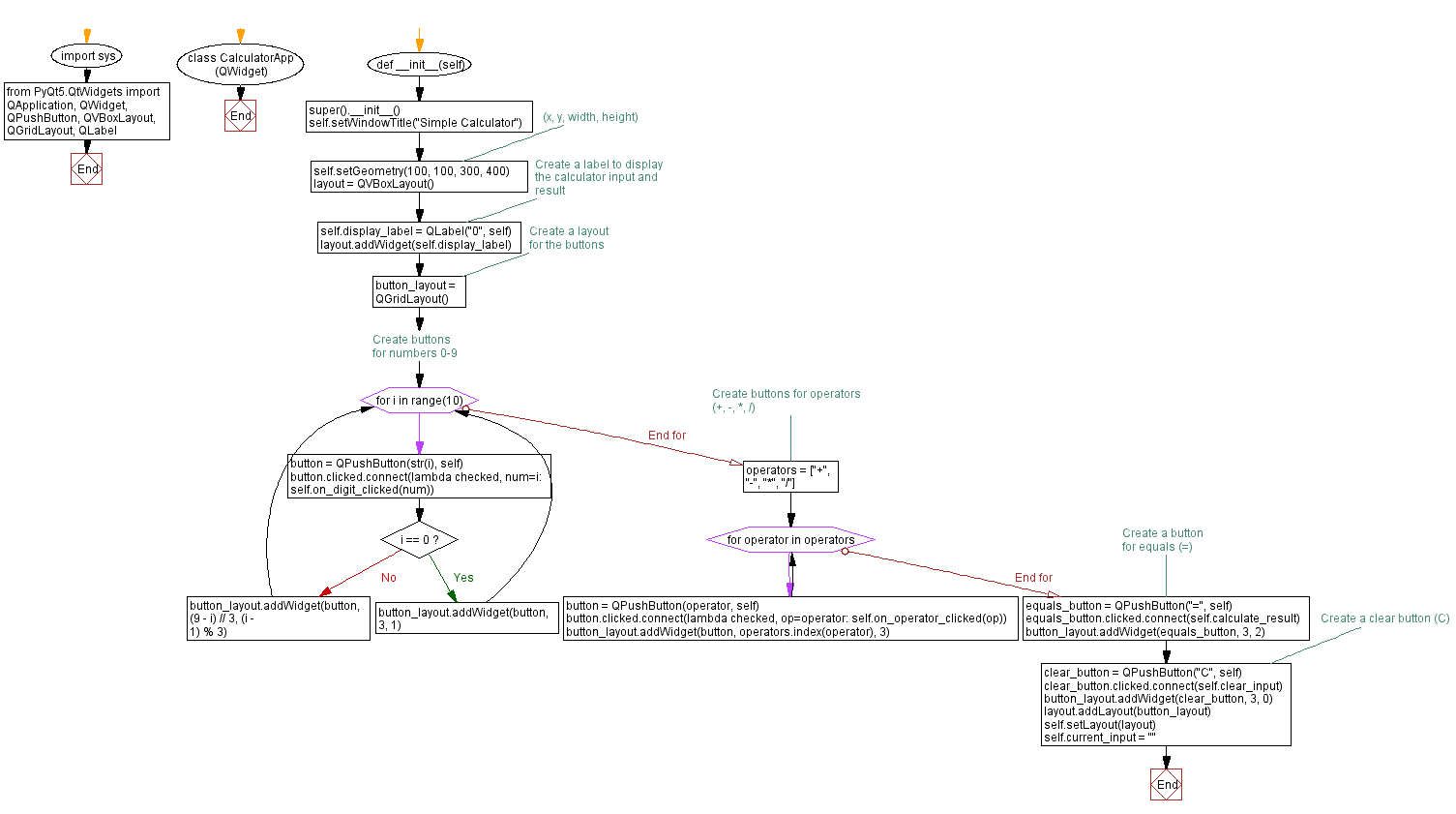
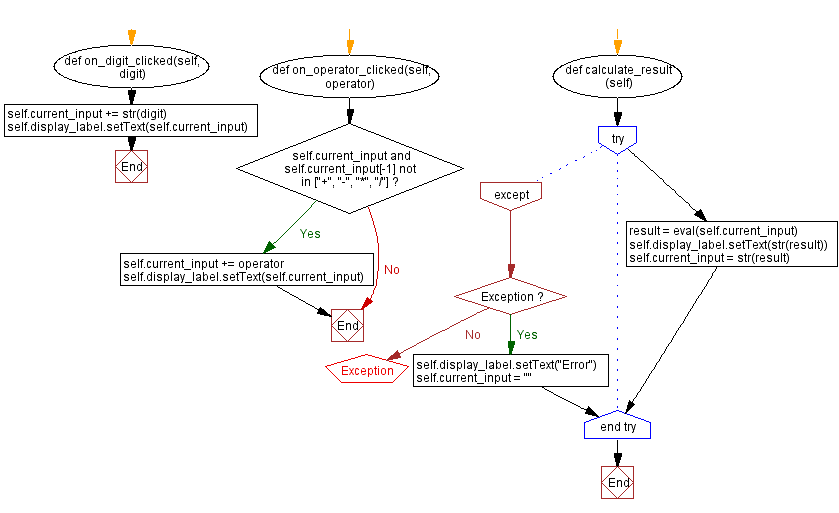
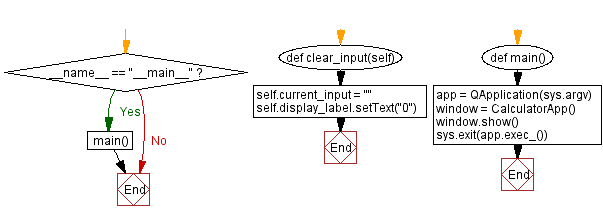
Go to:
Previous: Python PyQt character counter notepad.
Next: Python PyQt radio button example.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.