Python PyQt program - Display Hello, PyQt!
Write a Python program that displays the text "Hello, PyQt!" in the center of the window using PyQt.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QLabel Class: The QLabel widget provides a text or image display.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QWidget: The QWidget class is the base class of all user interface objects.
Qt module: PyQt5 is a set of Python bindings for the Qt application framework. It allows us to use Qt, a popular C++ framework, to create graphical user interfaces (GUIs) in Python.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QVBoxLayout, QWidget
from PyQt5.QtCore import Qt
class HelloPyQtApp(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Hello, PyQt!")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create a QLabel widget with the text "Hello, PyQt!"
label = QLabel("Hello, PyQt!")
# Create a layout for the central widget and add the label
layout = QVBoxLayout()
layout.addWidget(label)
# Center-align the label
label.setAlignment(Qt.AlignmentFlag.AlignCenter)
# Set the layout for the central widget
central_widget.setLayout(layout)
def main():
app = QApplication(sys.argv)
window = HelloPyQtApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a "QMainWindow" named HelloPyQtApp.
- Set the window's title and initial size using self.setWindowTitle and 'self.setGeometry'.
- Create a central widget using QWidget and set it as the central widget of the main window using 'self.setCentralWidget'.
- Create a "QLabel" widget named label with the text "Hello, PyQt!".
- Create a "QVBoxLayout" for the central widget, add the label to it, and center-align the label's text.
- In the main function, we set the layout for the central widget and execute the PyQt application's event loop.
Output:
Flowchart:
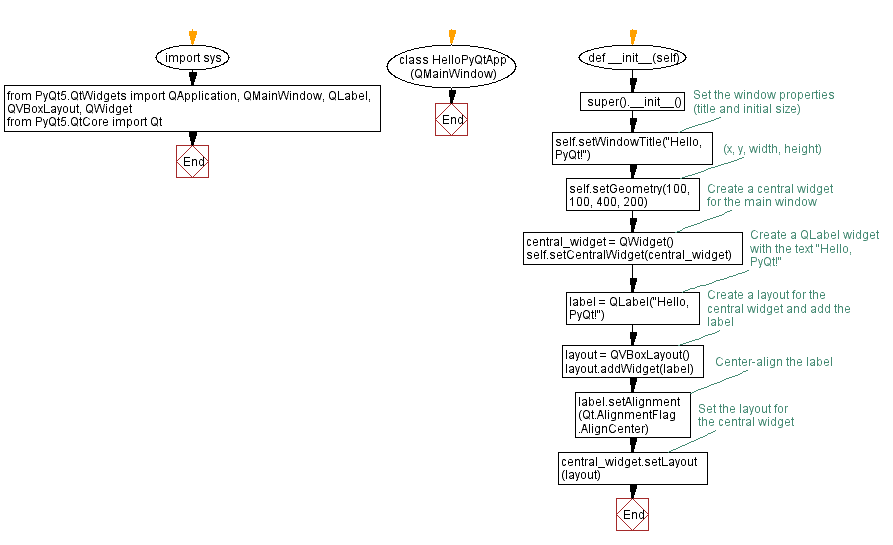
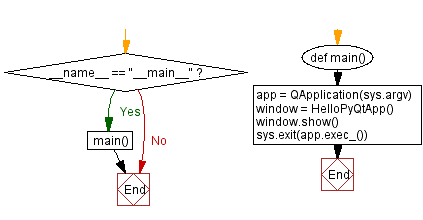
Go to:
Previous: Python PyQt Widgets Home.
Next: Yes or No buttons.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.