Python custom progress bar widget with PyQt
Write a Python program to create a custom progress bar widget that displays a progress percentage using PyQt. Create methods to set and update progress values.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QPushButton Class: The QPushButton widget provides a command button.
QPainter Class: The QPainter class performs low-level painting on widgets and other paint devices.
QColor Class: The QColor class provides colors based on RGB, HSV or CMYK values.
QFont Class: The QFont class specifies a query for a font used for drawing text.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QPushButton
from PyQt5.QtGui import QPainter, QColor, QFont
from PyQt5.QtCore import Qt, QTimer
class CustomProgressBar(QWidget):
def __init__(self):
super().__init__()
self.setMinimumSize(300, 50)
self.progress = 0
def set_progress(self, value):
self.progress = value
self.update()
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
# Define progress bar dimensions
bar_width = 250
bar_height = 30
bar_x = (self.width() - bar_width) / 2
bar_y = (self.height() - bar_height) / 2
# Draw the background of the progress bar
painter.setPen(Qt.NoPen)
painter.setBrush(QColor(200, 200, 200))
painter.drawRect(bar_x, bar_y, bar_width, bar_height)
# Draw the filled portion of the progress bar
fill_width = int(bar_width * (self.progress / 100))
painter.setBrush(QColor(0, 160, 230))
painter.drawRect(bar_x, bar_y, fill_width, bar_height)
# Display progress percentage text
font = QFont("Arial", 12)
painter.setFont(font)
painter.setPen(QColor(0, 0, 0))
text = f"{int(self.progress)}%"
text_width = painter.fontMetrics().width(text)
text_x = (self.width() - text_width) / 2
text_y = bar_y + bar_height + 20
painter.drawText(text_x, text_y, text)
class ProgressBarApp(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Custom Progress Bar")
self.setGeometry(100, 100, 400, 200)
layout = QVBoxLayout()
self.progress_bar = CustomProgressBar()
layout.addWidget(self.progress_bar)
self.button = QPushButton("Start Progress")
self.button.clicked.connect(self.start_progress)
layout.addWidget(self.button)
self.setLayout(layout)
# Initialize timer for smooth progress update
self.timer = QTimer(self)
self.timer.timeout.connect(self.update_progress)
def start_progress(self):
self.progress = 0
self.button.setEnabled(False)
self.timer.start(100) # Update every 100 milliseconds
def update_progress(self):
if self.progress <= 100:
self.progress_bar.set_progress(self.progress)
self.progress += 1
else:
self.timer.stop()
self.button.setEnabled(True)
def main():
app = QApplication(sys.argv)
window = ProgressBarApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a CustomProgressBar class that subclasses QWidget to create the custom progress bar widget.
- The "set_progress()" method allows you to set the progress value and triggers a repaint of the progress bar.
- In the "paintEvent()" method, we use QPainter to draw the progress bar and the filled portion based on the progress value.
- Display the progress percentage text below the progress bar.
- The "ProgressBarApp" class creates the main application window with a custom progress bar and a button to start progress updates.
- Added a QTimer named timer to the ProgressBarApp class. This timer periodically calls the "update_progress()" method.
- The "start_progress()" initializes the progress value and starts the timer to update the progress every 100 milliseconds.
- It is disabled during progress updates and enabled after completion.
Output:
Flowchart:
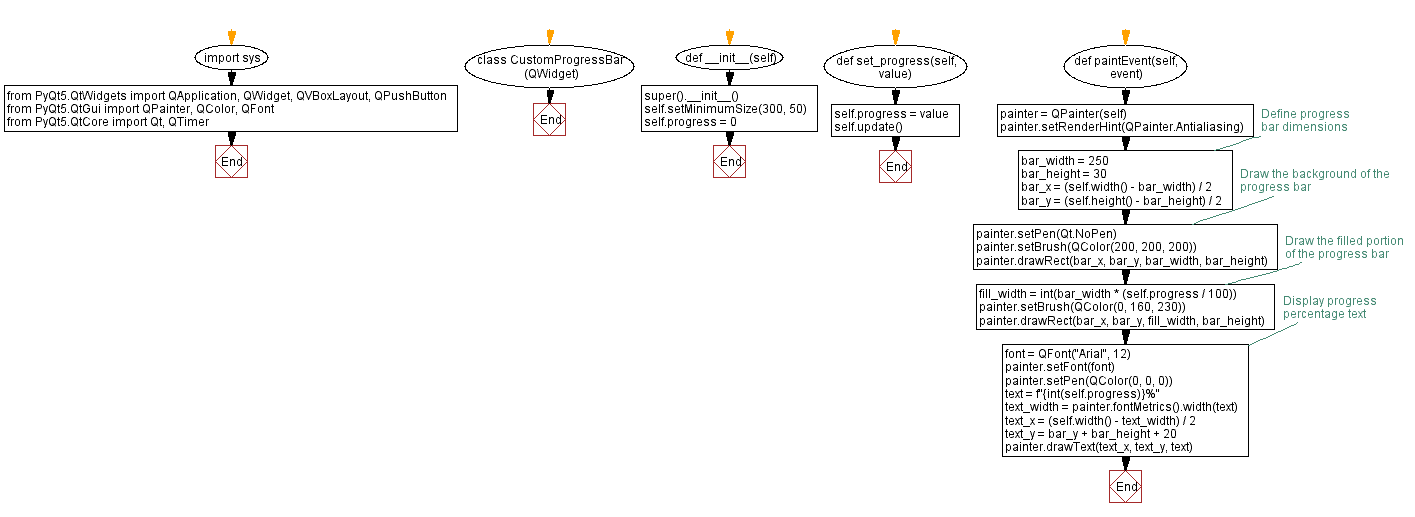
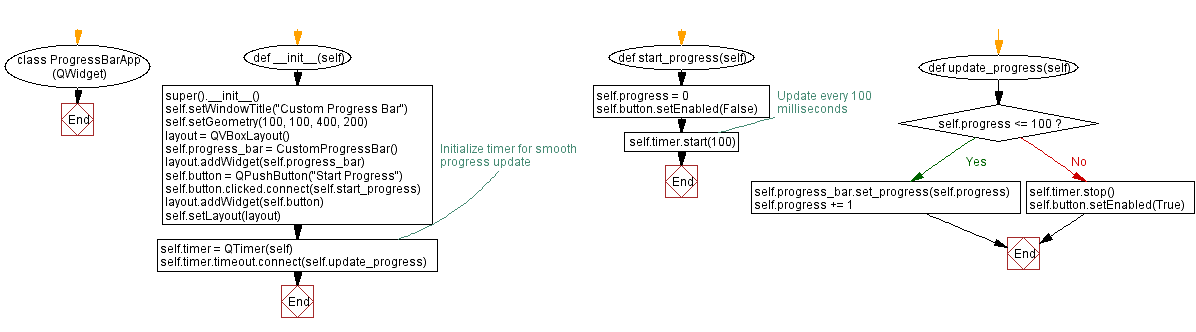
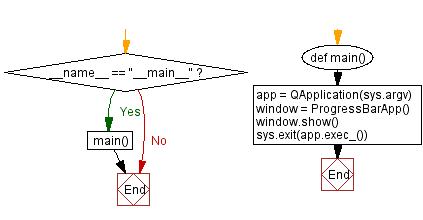
Go to:
Previous: Messaging interface.
Next: Python PyQt Connecting Signals to Slots Exercises Home.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.