Python PyQt program - Simple text editor
Write a Python program to create a simple text editor using PyQt. Allow users to input and save text to a file.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QTextEdit Class: The QTextEdit class provides a widget that is used to edit and display both plain and rich text.
QAction Class: The QAction class provides an abstraction for user commands that can be added to different user interface components.
QFileDialog Class: The QFileDialog class provides a dialog that allow users to select files or directories.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QWidget Class: The QWidget class is the base class of all user interface objects.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit, QAction, QFileDialog, QVBoxLayout, QWidget
class TextEditorApp(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Text Editor")
self.setGeometry(100, 100, 600, 400) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create a QTextEdit widget for text input
self.text_edit = QTextEdit()
self.text_edit.setFontPointSize(14) # Set font size
# Create actions for Open and Save
open_action = QAction("Open", self)
save_action = QAction("Save", self)
# Connect actions to methods
open_action.triggered.connect(self.open_file)
save_action.triggered.connect(self.save_file)
# Create a menu bar with File menu
menu_bar = self.menuBar()
file_menu = menu_bar.addMenu("File")
file_menu.addAction(open_action)
file_menu.addAction(save_action)
# Create a layout for the central widget and add the QTextEdit
layout = QVBoxLayout()
layout.addWidget(self.text_edit)
# Set the layout for the central widget
central_widget.setLayout(layout)
def open_file(self):
options = QFileDialog.Options()
options |= QFileDialog.ReadOnly # Open file in read-only mode
file_path, _ = QFileDialog.getOpenFileName(self, "Open File", "", "Text Files (*.txt);;All Files (*)", options=options)
if file_path:
with open(file_path, 'r') as file:
self.text_edit.setPlainText(file.read())
def save_file(self):
options = QFileDialog.Options()
options |= QFileDialog.ReadOnly # Open file in read-only mode
file_path, _ = QFileDialog.getSaveFileName(self, "Save File", "", "Text Files (*.txt);;All Files (*)", options=options)
if file_path:
with open(file_path, 'w') as file:
file.write(self.text_edit.toPlainText())
def main():
app = QApplication(sys.argv)
window = TextEditorApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a "QMainWindow" named 'TextEditorApp' with a central widget.
- Set the window's title and initial size.
- Create a "QTextEdit" widget (text_edit) for text input and customize its appearance by setting the font size.
- Create "Open" and "Save" actions and connect them to the open_file and save_file methods, respectively.
- Create a menu bar with a "File" menu that contains the "Open" and "Save" actions.
- The "open_file()" method opens a file dialog and loads the selected file's contents into QTextEdit.
- The "save_file()" method opens a file dialog and saves QTextEdit's contents to the selected file.
- In the main function, we create the PyQt application, create an instance of the "TextEditorApp" class, show the window, and run the application's event loop.
Output:
Flowchart:
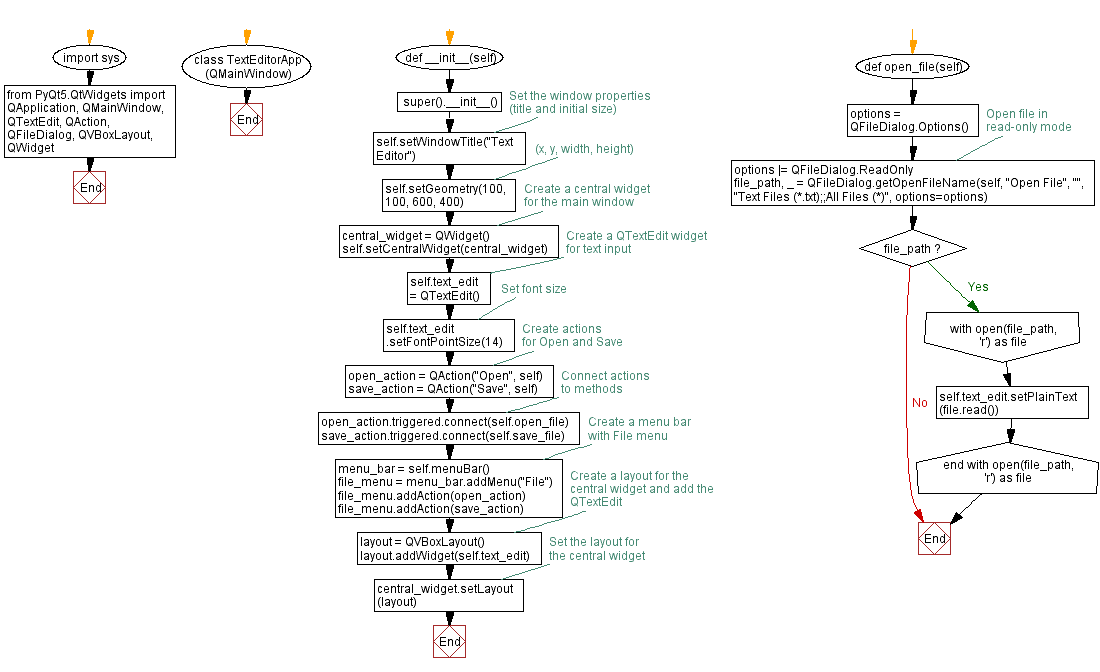
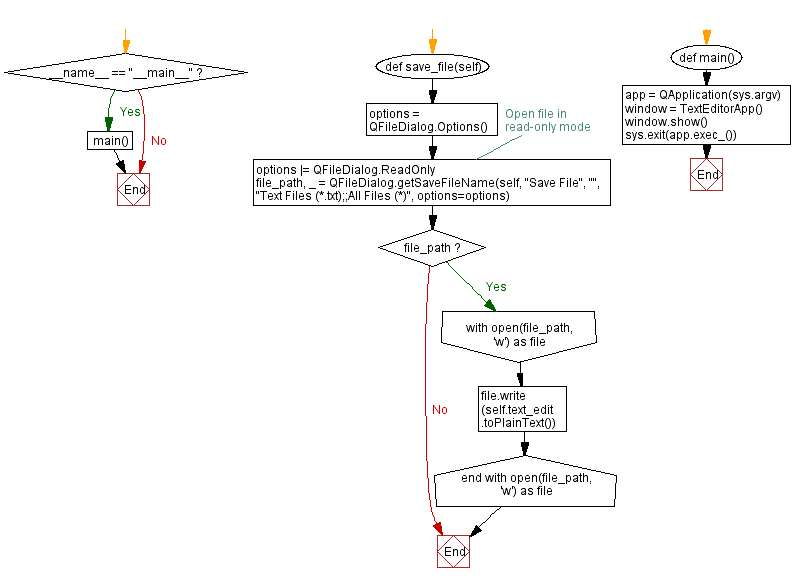
Go to:
Previous: Yes or No buttons.
Next: Color picker.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.