Python PyQt program - Button color changer
Write a Python program that designs a PyQt application with a push button that changes its text and background color when clicked.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QPushButton: The push button, or command button, is perhaps the most commonly used widget in any graphical user interface. Push (click) a button to command the computer to perform some action, or to answer a question. Typical buttons are OK, Apply, Cancel, Close, Yes, No and Help.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QWidget: The QWidget class is the base class of all user interface objects.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QVBoxLayout, QWidget
class ButtonColorChangerApp(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Button Color Changer")
self.setGeometry(100, 100, 300, 200) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create a QPushButton with an initial text
self.button = QPushButton("Click me to change the color and text!")
self.button.clicked.connect(self.change_button_color)
# Create a layout for the central widget and add the button
layout = QVBoxLayout()
layout.addWidget(self.button)
# Set the layout for the central widget
central_widget.setLayout(layout)
def change_button_color(self):
# Change the text and background color of the button
if self.button.text() == "Click me to change the color and text!":
self.button.setText("Clicked!")
self.button.setStyleSheet("background-color: green; color: white;")
else:
self.button.setText("Click me to change the color and text!")
self.button.setStyleSheet("background-color: blue; color: white;")
def main():
app = QApplication(sys.argv)
window = ButtonColorChangerApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a "QMainWindow" named 'ButtonColorChangerApp' with a central widget.
- Set the window's title and initial size.
- Create a QPushButton (button) with an initial text of "Click Me" and connect its clicked signal to the change_button_color method.
- When "change_button_color()" is called, the button's text and background color are toggled between two states.
- Set up the central widget layout and add the button to it.
- In the main() function, we create the PyQt application, create an instance of the "ButtonColorChangerApp" class, show the window, and run the application's event loop.
Output:
Flowchart:
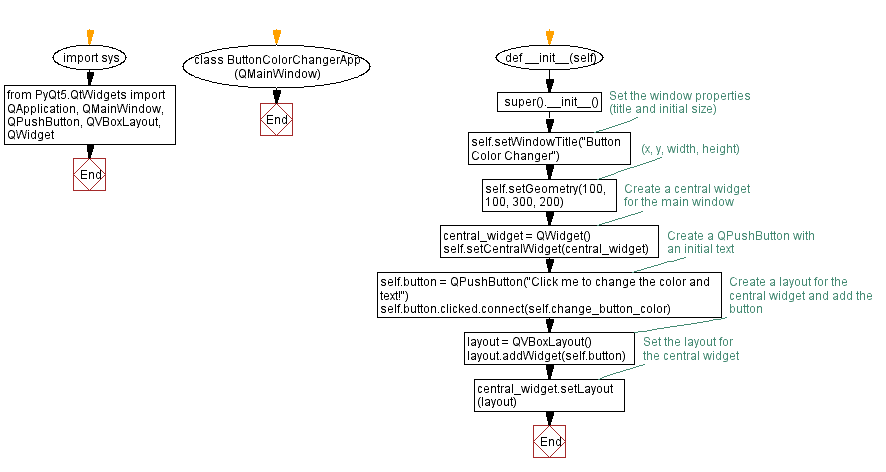
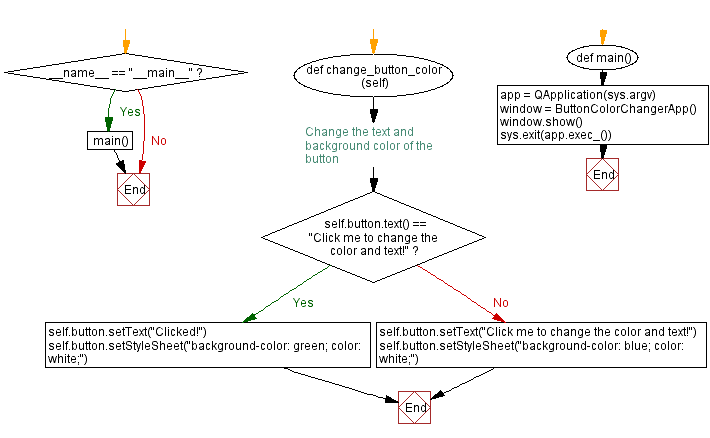
Go to:
Previous: Color picker.
Next: Customize appearance.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.