Python: Check if a number is positive, negative or zero
Python Basic: Exercise-109 with Solution
Write a Python program to check if a number is positive, negative or zero.
Positive Numbers: Any number above zero is known as a positive number. Positive numbers are written without any sign or a '+' sign in front of them and they are counted up from zero, i.e. 1, + 2, 3, +4 etc.
Negative Numbers: Any number below zero is known as a negative number. Negative numbers are always written with a '−' sign in front of them and they are counted down from zero, i.e. -1, -2, -3, -4 etc.
Always look at the sign in front of a number to check if it is positive or negative.
Zero, 0, is neither positive nor negative.
Pictorial Presentation:

Sample Solution-1:
Python Code:
# Prompt the user to input a number, and convert the input to a floating-point number.
num = float(input("Input a number: "))
# Check if the number is greater than zero.
if num > 0:
# If true, print that it is a positive number.
print("It is a positive number")
# Check if the number is equal to zero.
elif num == 0:
# If true, print that it is zero.
print("It is zero")
else:
# If the above conditions are not met, print that it is a negative number.
print("It is a negative number")
Sample Output:
Input a number: 150 It is positive number
Flowchart:
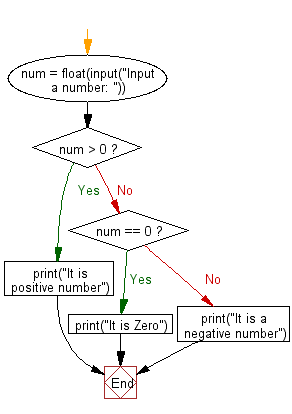
Sample Solution-2:
Python Code:
# Prompt the user to input a number and convert the input to a floating-point number.
n = float(input('Input a number: '))
# Use a conditional expression (ternary operator) to determine if the number is positive, zero, or negative.
# The conditional expression checks if the number is greater than 0, equal to 0, or less than 0.
# Depending on the condition met, it prints a corresponding message.
print('Number is Positive.' if n > 0 else 'It is Zero!' if n == 0 else 'Number is Negative.')
Sample Output:
Input a number: 0 It is Zero!
Sample Solution-3:
Python Code:
# Prompt the user to input a number and convert the input to a floating-point number.
n = float(input("Input a number: "))
# Check if the input number is greater than or equal to 0.
if n >= 0:
# If the number is zero, print that it is zero.
if n == 0:
print("It is Zero!")
# If the number is greater than zero, print that it is a positive number.
else:
print("Number is Positive number.")
# If the number is less than zero, print that it is a negative number.
else:
print("Number is Negative number.")
Sample Output:
Input a number: -150 Number is Negative number.
Python Code Editor:
Previous: Write a Python program to find path refers to a file or directory when you encounter a path name.
Next: Write a Python program to get numbers divisible by fifteen from a list using an anonymous function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-basic-exercise-109.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics