Python: Check whether multiple variables have the same value
Variable Equality Checker
Write a Python program to check whether multiple variables have the same value.
Pictorial Presentation:
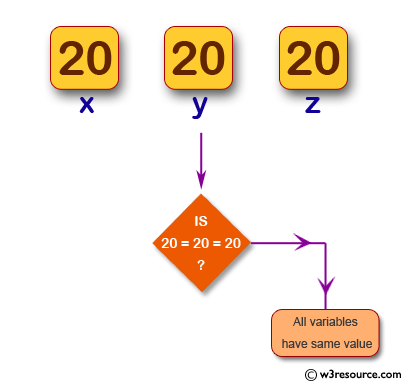
Sample Solution-1:
Python Code:
# Assign the value 20 to the variable x.
x = 20
# Assign the value 20 to the variable y.
y = 20
# Assign the value 20 to the variable z.
z = 20
# Check if all variables have the value 20 using the equality (==) operator.
if x == y == z == 20:
print("All variables have the same value!")
Sample Output:
All variables have same value!
Sample Solution-2:
Python Code:
# Define a function that checks if all variables passed as arguments have the same value.
def multiple_variables_equality(*vars):
# Iterate through the variables in the argument list.
for x in vars:
# Check if the current variable is not equal to the first variable.
if x != vars[0]:
return "All variables do not have the same value."
return "All variables have the same value."
# Call the function with different sets of variables and print the result.
print(multiple_variables_equality(2, 3, 2, 2, 2, 2))
print(multiple_variables_equality(10, 10, 10, 10))
print(multiple_variables_equality(-3, -3, -3, -3))
Sample Output:
All variables have not same value. All variables have same value. All variables have same value.
Flowchart:
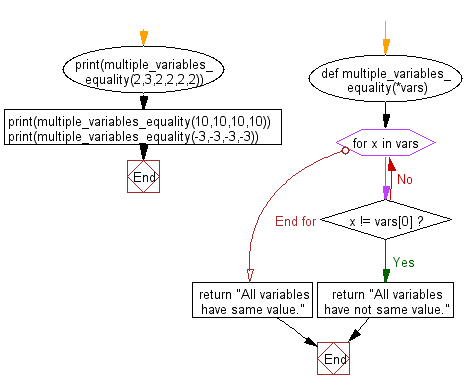
For more Practice: Solve these Related Problems:
- Write a Python program to check if three different variables reference the same memory location.
- Write a Python function to check if multiple variables have different values.
- Write a Python program that determines equality of variables when stored in different data structures.
- Write a Python program to check if two different variables hold the same mutable object.
Python Code Editor:
Previous: Write a Python program to determine the largest and smallest integers, longs, floats.
Next: Write a Python program to sum of all counts in a collections.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics