Python: Convert decimal to hexadecimal
Python Basic: Exercise-141 with Solution
Write a python program to convert decimal to hexadecimal.
Sample decimal number: 30, 4
Expected output: 1e, 04
Pictorial Presentation:
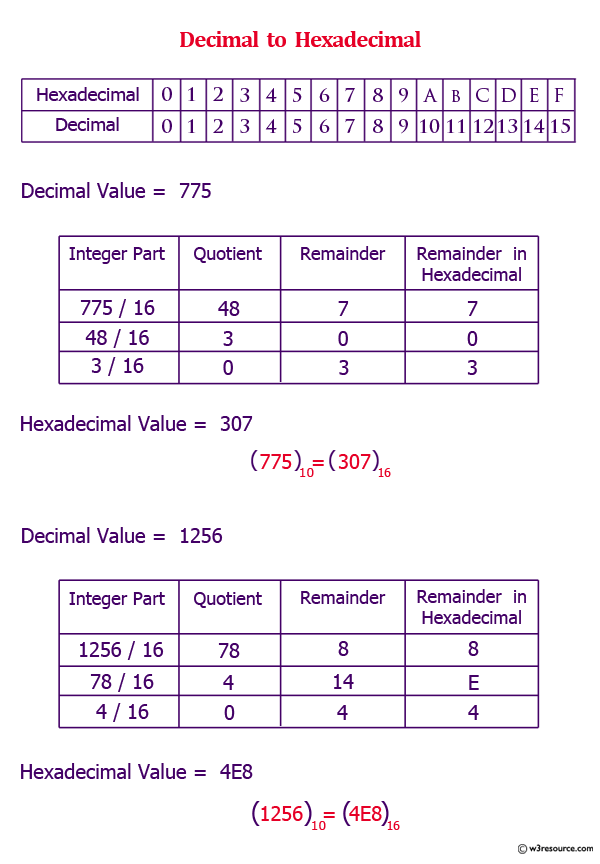
Sample Solution-1:
Python Code:
# Define an integer variable 'x' with the value 30.
x = 30
# Print the hexadecimal representation of 'x' with leading zeros.
# The 'format' function is used with the format specifier '02x' to format 'x' as a 2-character lowercase hexadecimal string.
# It ensures that there are leading zeros to make it 2 characters long.
print(format(x, '02x'))
# Define an integer variable 'x' with the value 4.
x = 4
# Print the hexadecimal representation of 'x' with leading zeros.
# The 'format' function is used with the format specifier '02x' to format 'x' as a 2-character lowercase hexadecimal string.
# It ensures that there are leading zeros to make it 2 characters long.
print(format(x, '02x'))
Sample Output:
1e 04
Sample Solution-2:
Python Code:
# Define a function 'dechimal_to_Hex' that converts a decimal number to hexadecimal.
# The function takes an integer 'n' as input.
def dechimal_to_Hex(n):
# Calculate the remainder when 'n' is divided by 16.
x = (n % 16)
# Initialize an empty string 'ch' to store the hexadecimal character.
ch = ""
# Check the value of 'x' to determine the corresponding hexadecimal character.
# For values 0-9, use the integer itself. For values 10-15, use the letters 'A' to 'F'.
if (x < 10):
ch = x
if (x == 10):
ch = "A"
if (x == 11):
ch = "B"
if (x == 12):
ch = "C"
if (x == 13):
ch = "D"
if (x == 14):
ch = "E"
if (x == 15):
ch = "F"
# Check if there are more digits in the number to convert.
if (n - x != 0):
# Recursively call 'dechimal_to_Hex' to convert the remaining digits and append the current hexadecimal character 'ch'.
return dechimal_to_Hex(n // 16) + str(ch)
else:
# If there are no more digits to convert, return the hexadecimal character 'ch'.
return str(ch)
# Define a list of decimal numbers 'dechimal_nums'.
dechimal_nums = [0, 15, 30, 55, 355, 656, 896, 1125]
# Print the original decimal numbers.
print("Decimal numbers:")
print(dechimal_nums)
# Convert and print the hexadecimal representations of the decimal numbers using the 'dechimal_to_Hex' function.
print("\nHexadecimal numbers of the said decimal numbers:")
print([dechimal_to_Hex(x) for x in dechimal_nums])
Sample Output:
Dechimal numbers: [0, 15, 30, 55, 355, 656, 896, 1125] Hexadechimal numbers of the said dechimal numbers: ['0', 'F', '1E', '37', '163', '290', '380', '465']
Flowchart:
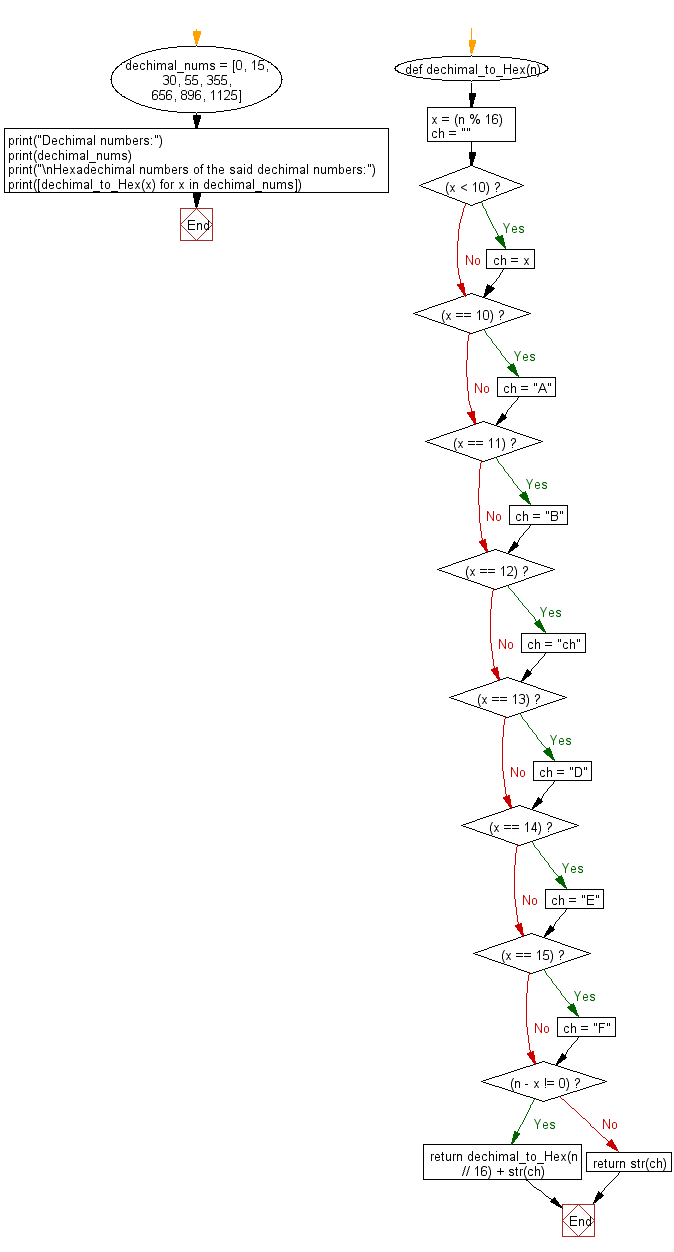
Sample Solution-3:
Python Code:
# Define a function 'dechimal_to_Hex' that converts a list of decimal numbers to hexadecimal.
# The function takes a list of decimal numbers 'dechimal_nums' as input.
def dechimal_to_Hex(dechimal_nums):
# Define a string 'digits' containing hexadecimal digits.
digits = "0123456789ABCDEF"
# Initialize 'x' with the remainder when 'dechimal_nums' is divided by 16.
x = (dechimal_nums % 16)
# Calculate the integer division of 'dechimal_nums' by 16 and store it in 'rest_part'.
rest_part = dechimal_nums // 16
# Check if 'rest_part' is zero.
if (rest_part == 0):
# If there is no remainder, return the hexadecimal digit corresponding to 'x'.
return digits[x]
# If 'rest_part' is not zero, recursively call 'dechimal_to_Hex' with the 'rest_part' and append the hexadecimal digit corresponding to 'x'.
return dechimal_to_Hex(rest_part) + digits[x]
# Define a list of decimal numbers 'dechimal_nums'.
dechimal_nums = [0, 15, 30, 55, 355, 656, 896, 1125]
# Print the original decimal numbers.
print("Decimal numbers:")
print(dechimal_nums)
# Convert and print the hexadecimal representations of the decimal numbers using the 'dechimal_to_Hex' function.
print("\nHexadecimal numbers of the said decimal numbers:")
print([dechimal_to_Hex(x) for x in dechimal_nums])
Sample Output:
Dechimal numbers: [0, 15, 30, 55, 355, 656, 896, 1125] Hexadechimal numbers of the said dechimal numbers: ['0', 'F', '1E', '37', '163', '290', '380', '465']
Flowchart:
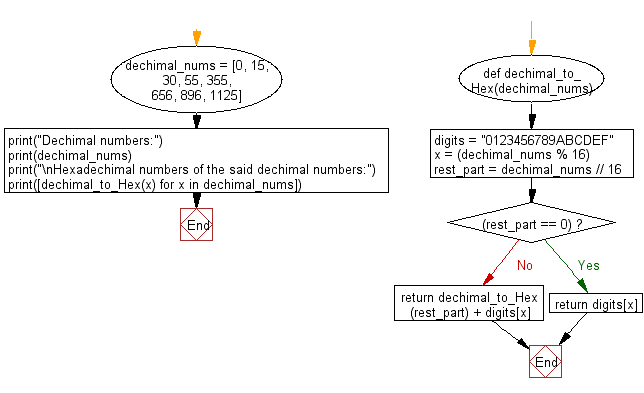
Python Code Editor:
Previous: Write a Python program to convert an integer to binary keep leading zeros.
Next: Write a Python program to check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones of same length in a given string. Return True/False.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-basic-exercise-141.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics