Python: Check whether variable is integer or string
Variable Type Checker
Write a Python program to check whether a variable is an integer or string.
Sample Solution-1:
Python Code :
# Check if 25 is an instance of an integer (int) or a string (str).
print(isinstance(25, int) or isinstance(25, str))
# Check if the list containing 25 is an instance of an integer (int) or a string (str).
print(isinstance([25], int) or isinstance([25], str))
# Check if the string "25" is an instance of an integer (int) or a string (str).
print(isinstance("25", int) or isinstance("25", str))
Sample Output:
True False True
Flowchart:
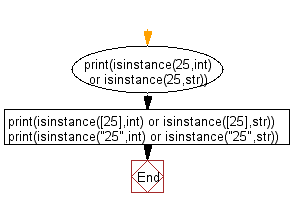
Sample Solution-2:
Python Code :
# Define a function 'test' that takes a parameter 'n'.
def test(n):
# Check if the type of 'n' is equal to 'int'.
if type(n) == int:
# If 'n' is an integer, return the message "It is an integer!"
return "It is an integer!"
else:
# If 'n' is not an integer, return the message "It is a String!"
return "It is a String!"
# Call the 'test' function with an integer argument (12) and print the result.
print(test(12))
# Call the 'test' function with a string argument ('23312') and print the result.
print(test('23312'))
Sample Output:
It is an integer! It is a String!
Flowchart:
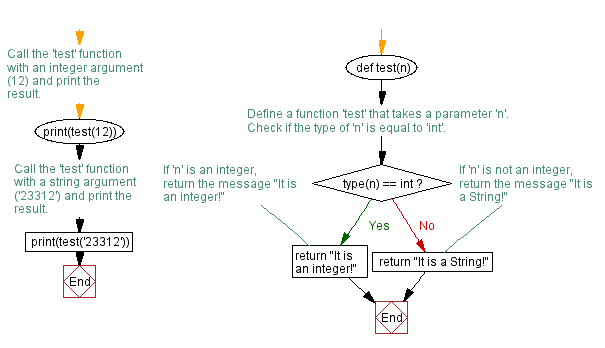
For more Practice: Solve these Related Problems:
- Write a Python function to determine if a variable is a float or a complex number.
- Write a Python program to check if a given variable is a dictionary or a list.
- Write a Python function to check if a variable is of a user-defined class type.
- Write a Python program to check if a variable is immutable.
Python Code Editor:
Previous: Write a Python program to determine whether the python shell is executing in 32bit or 64bit mode on operating system.
Next: Write a Python program to test whether a variable is a list or tuple or a set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics