Python: Calculate volume of sphere
Python Basic: Exercise-15 with Solution
Write a Python program to get the volume of a sphere with radius six.
Python: Volume of a Sphere
A sphere is a three-dimensional solid with no face, no edge, no base and no vertex. It is a round body with all points on its surface equidistant from the center. The volume of a sphere is measured in cubic units.
The volume of the sphere is : V = 4/3 × π × r3 = π × d3/6.
Sample Solution:
Python Code:
# Define the value of pi
pi = 3.1415926535897931
# Define the radius of the sphere
r = 6.0
# Calculate the volume of the sphere using the formula
V = 4.0/3.0 * pi * r**3
# Print the calculated volume of the sphere
print('The volume of the sphere is: ', V)
Sample Output:
The volume of the sphere is: 904.7786842338603
Explanation:
The said code assigns the value of pi as 3.1415926535897931 to the variable "pi" and 6.0 to the variable "r". The code uses the formula V = 4/3 * pi * r^3 to calculate the volume of a sphere. Volume of the sphere is stored in the variable "V".
It then prints the message "The volume of the sphere is:" followed by the volume of the sphere using print statement.
In this case, the code will output "The volume of the sphere is: 904.7786842338603".
Flowchart:
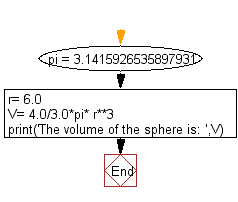
Python Code Editor:
Previous: Write a Python program to calculate number of days between two dates.
Next: Write a Python program to get the difference between a given number and 17, if the number is greater than 17 return double the absolute difference.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-basic-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics