Python: Check whether a specified value is contained in a group of values
Value in Group Tester
Write a Python program that checks whether a specified value is contained within a group of values.
Test Data:
3 -> [1, 5, 8, 3] : True
-1 -> [1, 5, 8, 3] : False
Pictorial Presentation:
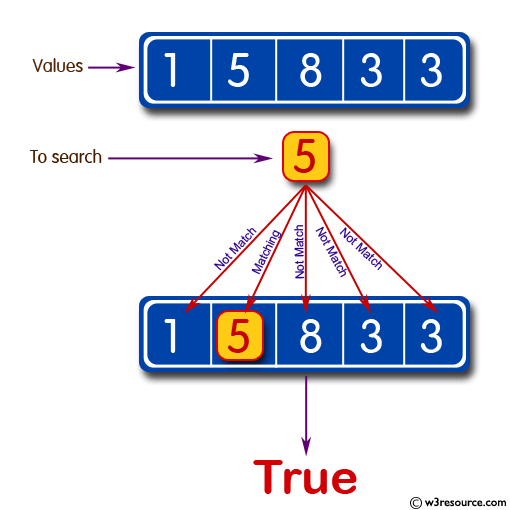
Sample Solution-1:
Python Code:
# Define a function called is_group_member that takes two parameters: group_data (a list) and n (an integer).
def is_group_member(group_data, n):
# Iterate through the elements (values) in the group_data list.
for value in group_data:
# Check if the current value is equal to the given integer, n.
if n == value:
return True # If found, return True.
return False # If the loop completes and no match is found, return False.
# Call the is_group_member function with two different lists and integers, and print the results.
print(is_group_member([1, 5, 8, 3], 3)) # Output: True (3 is in the list)
print(is_group_member([5, 8, 3], -1)) # Output: False (-1 is not in the list)
Sample Output:
True False
Explanation:
The said code defines a function called "is_group_member" that takes two arguments "group_data" a list and an integer "n". First, the function declares a for loop to iterate through each element in the "group_data" list. When the current element (value) value matches the value of "n", it returns True, which means the input integer is present in the "group_data" list. The function returns False if the for loop fails to find a match, meaning the input integer does not exist in "group_data".
In the last two lines the code call the function "is_group_member" twice, with the input values ([1, 5, 8, 3], 3) and ([5, 8, 3], -1) and print the results.
In the first case, False will be returned, while in the second case, True will be returned.
Flowchart:
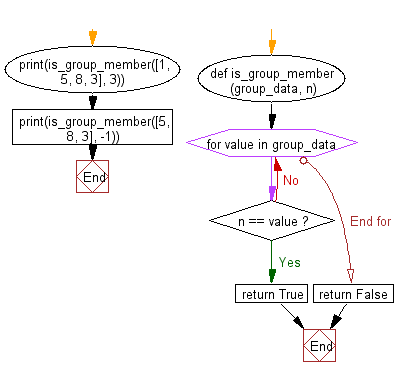
Sample Solution-2:
Python Code:
# Define a function called is_group_member that takes two parameters: group_data (a list) and n (an integer).
def is_group_member(group_data, n):
return n in group_data # Check if n is in the group_data list and return the result.
# Call the is_group_member function with two different lists and integers and print the results.
print(is_group_member([1, 5, 8, 3], 3)) # Output: True (3 is in the list)
print(is_group_member([5, 8, 3], -1)) # Output: False (-1 is not in the list)
Sample Output:
True False
Explanation:
The said code defines a function called "is_group_member" which takes two parameters: "group_data", a list and "n", an integer. Within the function, it uses the "in" operator to check if the variable "n" is present in the "group_data" passed as an argument. If it is present, the function returns true, otherwise false.
The code then calls the function twice, passing two different arguments, ([1, 5, 8, 3], 3) and ([5, 8, 3], -1) and print the results.
It prints the output of the function which is True and False respectively.
Flowchart:
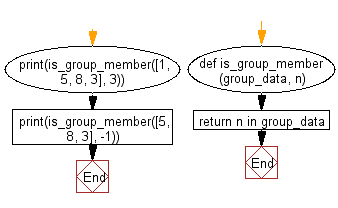
For more Practice: Solve these Related Problems:
- Write a Python program to check if multiple given values exist in a list.
- Write a script to check if all elements in a list are within a given range.
- Write a function that finds the first occurrence of a specified value in a list and returns its index.
- Write a program that determines whether any element in one list is present in another list.
Go to:
Previous: Write a Python program to test whether a passed letter is a vowel or not.
Next: Write a Python program to create a histogram from a given list of integers.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.