Python: Print out a set containing all the colors from a list which are not present in other list
Python Basic: Exercise-29 with Solution
Write a Python program that prints out all colors from color_list_1 that are not present in color_list_2.
Test Data:
color_list_1 = set(["White", "Black", "Red"])
color_list_2 = set(["Red", "Green"])
Expected Output :
{'Black', 'White'}
Pictorial Presentation:
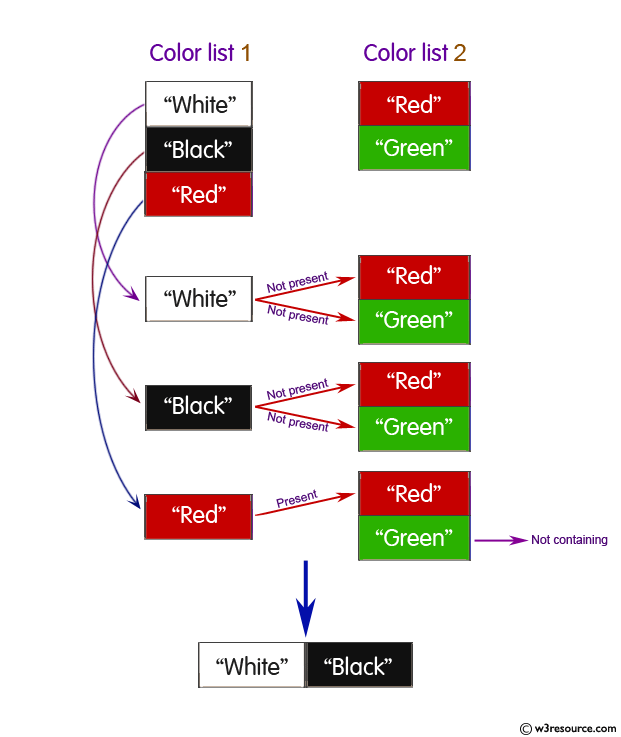
Sample Solution-1:
Python Code:
# Create two sets, color_list_1 and color_list_2.
color_list_1 = set(["White", "Black", "Red"])
color_list_2 = set(["Red", "Green"])
# Print the original elements of the sets.
print("Original set elements:")
print(color_list_1)
print(color_list_2)
# Calculate and print the difference of color_list_1 and color_list_2.
print("\nDifference of color_list_1 and color_list_2:")
print(color_list_1.difference(color_list_2))
# Calculate and print the difference of color_list_2 and color_list_1.
print("\nDifference of color_list_2 and color_list_1:")
print(color_list_2.difference(color_list_1))
Sample Output:
Original set elements: {'White', 'Black', 'Red'} {'Green', 'Red'} Differenct of color_list_1 and color_list_2: {'White', 'Black'} Differenct of color_list_2 and color_list_1: {'Green'}
Explanation:
The said code defines two sets called "color_list_1" and "color_list_2" containing string elements.
The elements of both sets are then printed.
After that, it uses the difference() method on "color_list_1" and passes "color_list_2" as an argument to it. This method returns the elements that are in the "color_list_1" but not in "color_list_2".
Again it uses the difference() method on "color_list_2" and passes "color_list_1" as an argument to it. This method returns the elements that are in the "color_list_2" but not in "color_list_1".
The output of the code will be:
Original set elements:
{'Red', 'White', 'Black'}
{'Red', 'Green'}
Differenct of color_list_1 and color_list_2:
{'White', 'Black'}
Differenct of color_list_2 and color_list_1:
{'Green'}
Sample Solution-2:
Python Code:
# Create two sets, color_list_1 and color_list_2.
color_list_1 = set(["White", "Black", "Red"])
color_list_2 = set(["Red", "Green"])
# Print the original elements of the sets.
print("Original set elements:")
print(color_list_1)
print(color_list_2)
# Calculate and print the difference of color_list_1 and color_list_2 using set difference (-) operator.
print("\nDifference of color_list_1 and color_list_2:")
print(color_list_1 - color_list_2)
# Calculate and print the difference of color_list_2 and color_list_1 using set difference (-) operator.
print("\nDifference of color_list_2 and color_list_1:")
print(color_list_2 - color_list_1)
Sample Output:
Original set elements: {'Red', 'Black', 'White'} {'Red', 'Green'} Differenct of color_list_1 and color_list_2: {'Black', 'White'} Differenct of color_list_2 and color_list_1: {'Green'}
Explanation:
The said code defines two sets, "color_list_1" and "color_list_2", with the initial elements "White", "Black", "Red" and "Red", "Green" respectively.
It then prints the original sets, followed by the difference between color_list_1 and color_list_2 (the elements in color_list_1 that are not in color_list_2) and the difference between color_list_2 and color_list_1 (the elements in color_list_2 that are not in color_list_1).
Here the "-" operator is used to get the difference between two sets.
Python Code Editor:
Previous: Write a Python program to print out all even numbers from a given numbers list in the same order and stop the printing if any numbers that come after 237 in the sequence.
Next: Write a Python program that will accept the base and height of a triangle and compute the area.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-basic-exercise-29.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics