Python: How to find the distance between two points in Python?
Python Basic: Exercise-40 with Solution
Write a Python program to calculate the distance between the points (x1, y1) and (x2, y2).
Pictorial Presentation:
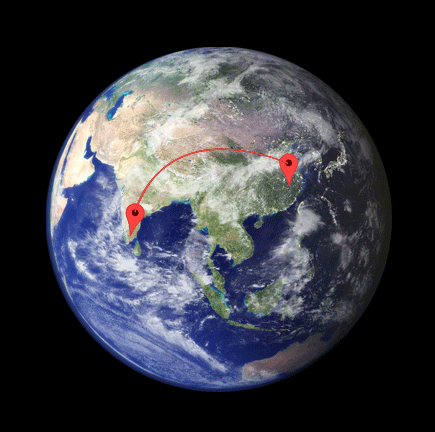
Sample Solution:
Python Code:
# Import the math module to use the square root function.
import math
# Define the coordinates of the first point (p1) as a list.
p1 = [4, 0]
# Define the coordinates of the second point (p2) as a list.
p2 = [6, 6]
# Calculate the distance between the two points using the distance formula.
# The formula computes the Euclidean distance in a 2D space.
distance = math.sqrt(((p1[0] - p2[0]) ** 2) + ((p1[1] - p2[1]) ** 2))
# Print the calculated distance.
print(distance)
Sample Output:
6.324555320336759
Explanation:
The said code calculates the Euclidean distance between two points in a 2-dimensional coordinate system.
- It starts by importing the "math" module which is used to calculate the square root of the given expression.
- Then it defines two variables p1 and p2 which are lists containing the x and y coordinates of two points. In this case, the first point is at coordinates (4, 0) and the second point is at coordinates (6, 6).
- The variable "distance" is defined and assigned the value of the Euclidean distance between the two points.
- The final distance is then printed using the print() function.
The final output will be 6.324555320336759 which is the distance between the two points (4,0) and (6,6).
Flowchart:
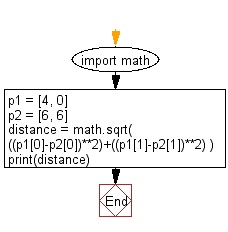
Python Code Editor:
Previous: Write a Python program to compute the future value of a specified principal amount, rate of interest, and a number of years.
Next: Write a Python program to check whether a file exists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-basic-exercise-40.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics