Python: Convert seconds to day, hour, minutes and seconds
Seconds to DHMS Converter
Write a Python program that converts seconds into days, hours, minutes, and seconds.
Pictorial Presentation:
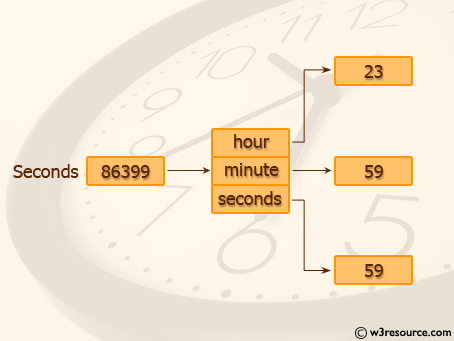
Sample Solution:
Python Code:
# Prompt the user to input a time duration in seconds and convert it to a float.
time = float(input("Input time in seconds: "))
# Calculate the number of full days in the given time duration.
day = time // (24 * 3600)
# Update the time variable to hold the remaining seconds after subtracting full days.
time = time % (24 * 3600)
# Calculate the number of full hours in the remaining time.
hour = time // 3600
# Update the time variable to hold the remaining seconds after subtracting full hours.
time %= 3600
# Calculate the number of full minutes in the remaining time.
minutes = time // 60
# Update the time variable to hold the remaining seconds after subtracting full minutes.
time %= 60
# The 'time' variable now represents the remaining seconds, which is the number of seconds.
seconds = time
# Print the time duration in the format "d:h:m:s".
print("d:h:m:s-> %d:%d:%d:%d" % (day, hour, minutes, seconds))
Sample Output:
Input time in seconds: 1234565 d:h:m:s-> 14:6:56:5
Flowchart:
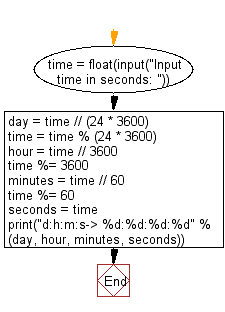
For more Practice: Solve these Related Problems:
- Write a Python program to convert a given number of milliseconds into days, hours, minutes, and seconds.
- Write a Python program to display the current system uptime in days, hours, minutes, and seconds.
- Write a Python program to calculate the total seconds in a given number of years.
- Write a Python program to convert a given number of nanoseconds into human-readable time.
Go to:
Previous: Write a Python program to get file creation and modification date/times.
Next: Write a Python program to calculate body mass index.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.