Python: Get a directory listing sorted by creation date
Directory Listing by Creation Date
Write a Python program to get a directory listing, sorted by creation date.
Sample Solution-1:
Python Code:
# Import necessary modules: 'stat' for file status, 'os' for operating system interactions,
# 'sys' for command-line arguments, and 'time' for time-related functions.
from stat import S_ISREG, ST_CTIME, ST_MODE
import os, sys, time
# Determine the directory path based on command-line arguments. If there are two arguments, use the second one as the path; otherwise, use the current directory.
dir_path = sys.argv[1] if len(sys.argv) == 2 else r'.'
# Generate a generator expression that yields the full path for each file in the specified directory.
data = (os.path.join(dir_path, fn) for fn in os.listdir(dir_path))
# Generate a generator expression that pairs the file's status information and its path.
data = ((os.stat(path), path) for path in data)
# Filter the files to keep only regular files (S_ISREG) and extract their creation times (ST_CTIME).
data = ((stat[ST_CTIME], path) for stat, path in data if S_ISREG(stat[ST_MODE]))
# Sort the files based on their creation times and then print the sorted list, including the creation time and the file name.
for cdate, path in sorted(data):
print(time.ctime(cdate), os.path.basename(path))
Sample Output:
Mon Feb 22 16:11:49 2016 .bash_logout Mon Feb 22 16:11:49 2016 .bashrc Mon Feb 22 16:11:49 2016 .profile Mon May 30 11:45:34 2016 .mysql_history Sat Aug 13 11:37:48 2016 logging_example.out Tue Sep 13 10:56:31 2016 result.txt Tue Sep 20 18:00:14 2016 dddd.txt ------- Tue Apr 18 15:06:27 2017 abc.txt Wed Apr 19 13:46:47 2017 .bash_history Wed Apr 19 15:15:52 2017 test.txt Wed Apr 19 16:58:20 2017 4ab8fe20-24f3-11e7-afe4-85767fd0ee52.py
Flowchart:
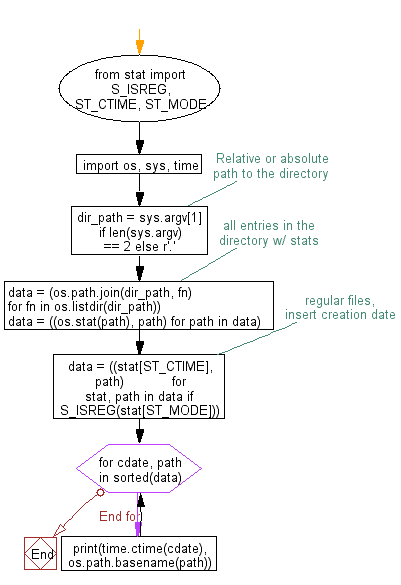
Sample Solution-2:
Python Code:
# Import the required modules: 'os' for operating system interactions and 'time' for time-related functions.
import os
import time
# Create a list 'paths' by iterating through files in the current directory and formatting their creation time and file name.
# The list comprehension generates tuples with the creation time and file name for each file.
paths = ["%s %s" % (time.ctime(t), f) for t, f in sorted([(os.path.getctime(x), x) for x in os.listdir(".")])]
# Print a header for the directory listing.
print("Directory listing, sorted by creation date:")
# Iterate through the list 'paths' and print each entry.
for x in range(len(paths)):
print(paths[x],)
Sample Output:
Directory listing, sorted by creation date: Mon May 31 13:29:45 2021 main.py
Flowchart:
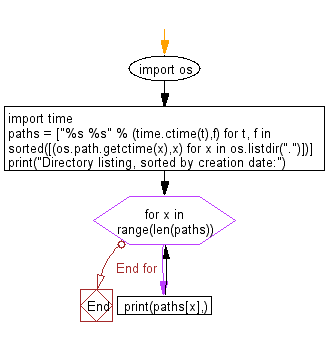
For more Practice: Solve these Related Problems:
- Write a Python program to list all directories inside a given folder sorted by creation date.
- Write a Python program to list files that were created within the last 7 days.
- Write a Python program to group files by creation year and month.
- Write a Python program to display files with their creation timestamps in descending order.
Python Code Editor:
Previous: Write a Python program to sort files by date.
Next: Write a Python program to get the details of math module.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics