Python: Command line arguments
Command-line Arguments
Write a Python program to get the command-line arguments (name of the script, the number of arguments, arguments) passed to a script.
Sample Solution:
Python Code (test.py):
# Import the sys module to access command-line arguments and other system-specific functionality.
import sys
# Display the message "This is the name/path of the script:" and print the script's name or path.
print("This is the name/path of the script:"), sys.argv[0]
# Display the message "Number of arguments:" and print the total number of command-line arguments.
print("Number of arguments:", len(sys.argv))
# Display the message "Argument List:" and print the list of command-line arguments passed to the script.
print("Argument List:", str(sys.argv))
The command executed in command prompt:
prashanta@server:~$ python test.py arg1 arg2 arg3
Sample Output:
This is the name/path of the script: test.py ('Number of arguments:', 4) ('Argument List:', "['test.py', 'arg1', 'arg2', 'arg3']")
Flowchart:
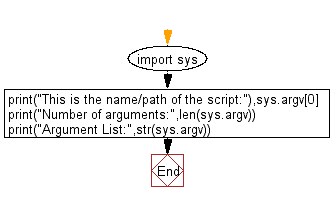
For more Practice: Solve these Related Problems:
- Write a Python program to count the number of command-line arguments passed to a script.
- Write a Python program to check if a specific argument exists in the command-line input.
- Write a Python program to accept multiple file names as command-line arguments and display their content.
- Write a Python program to validate numerical command-line arguments and perform a mathematical operation.
Python Code Editor:
-->
Previous: Write a Python program to get the copyright information and write Copyright information in Python code.
Next: Write a Python program to test whether the system is a big-endian platform or little-endian platform.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics