Python: Count the number of occurrence of a specific character in a string
Character Frequency Counter
Write a Python program to count the number of occurrences of a specific character in a string.
Pictorial Presentation:
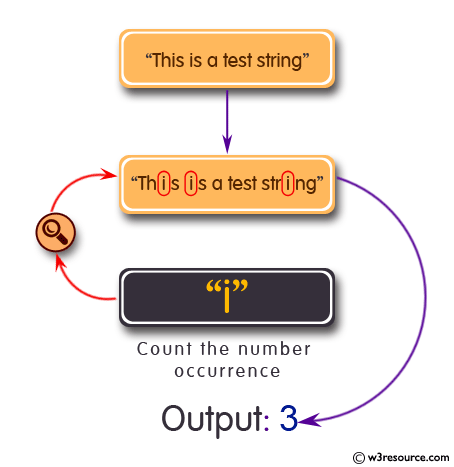
Sample Solution:
Using count() function:
Python Code:
# Create a string 's' containing a sentence.
s = "The quick brown fox jumps over the lazy dog."
# Print a message indicating the original string.
print("Original string:")
# Print the original string 's'.
print(s)
# Print a message indicating the number of occurrences of 'o' in the string.
print("Number of occurrences of 'o' in the said string:")
# Print the count of occurrences of the character 'o' in the string 's'.
print(s.count("o"))
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Number of occurrence of 'o' in the said string: 4
Using loop:
Python Code:
# Create a string 's' containing a sentence.
s = "The quick brown fox jumps over the lazy dog."
# Print a message indicating the original string.
print("Original string:")
# Print the original string 's'.
print(s)
# Print a message indicating the number of occurrences of 'o' in the string.
print("Number of occurrences of 'o' in the said string:")
# Initialize a counter variable 'ctr' to 0.
ctr = 0
# Iterate through each character 'c' in the string 's'.
for c in s:
# Check if the character 'c' is equal to 'o'.
if c == 'o':
# If 'c' is 'o', increment the counter 'ctr' by 1.
ctr = ctr + 1
# Print the final count of occurrences of 'o' in the string 's'.
print(ctr)
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Number of occurrence of 'o' in the said string: 4
Flowchart:
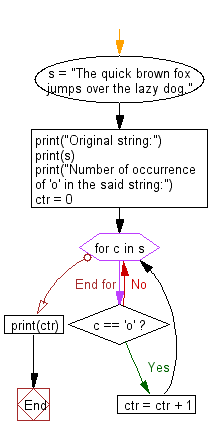
Using collections.Counter():
Python Code:
# Import the Counter class from the collections module.
from collections import Counter
# Create a string 's' containing a sentence.
s = "The quick brown fox jumps over the lazy dog."
# Print a message indicating the original string.
print("Original string:")
# Print the original string 's'.
print(s)
# Print a message indicating the number of occurrences of 'o' in the string.
print("Number of occurrences of 'o' in the said string:")
# Use the Counter class to count the occurrences of characters in the string 's'.
ctr = Counter(s)
# Print the count of the character 'o' from the Counter.
print(str(ctr['o']))
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Number of occurrence of 'o' in the said string: 4
Using Lambda functions:
Python Code:
# Create a string 's' containing a sentence.
s = "The quick brown fox jumps over the lazy dog."
# Print a message indicating the original string.
print("Original string:")
# Print the original string 's'.
print(s)
# Print a message indicating the number of occurrences of 'o' in the string.
print("Number of occurrences of 'o' in the said string:")
# Use the `map` function along with a lambda function to count occurrences of 'o' in the string 's'.
# It maps '1' for each character containing 'o' and '0' otherwise and then sums those values.
ctr = sum(map(lambda x: 1 if 'o' in x else 0, s))
# Print the count of occurrences of 'o'.
print(ctr)
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Number of occurrence of 'o' in the said string: 4
Using Regular Expressions:
Python Code:
# Import the 're' module, which provides support for regular expressions.
import re
# Create a string 's' containing a sentence.
s = "The quick brown fox jumps over the lazy dog."
# Print a message indicating the original string.
print("Original string:")
# Print the original string 's'.
print(s)
# Print a message indicating the number of occurrences of 'o' in the string.
print("Number of occurrences of 'o' in the said string:")
# Use the 're.findall' function to find all occurrences of 'o' in the string 's' and then count them.
ctr = len(re.findall("o", s))
# Print the count of occurrences of 'o'.
print(ctr)
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Number of occurrence of 'o' in the said string: 4
Python Code Editor:
Previous: Write a Python program to test if a certain number is greater than all numbers of a list.
Next: Write a Python program to check if a file path is a file or a directory.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics