Python: Create a copy of its own source code
Python Basic: Exercise-90 with Solution
Write a Python program to create a copy of its own source code.
Sample Solution:
Python Code:
# Define a function named file_copy that takes two arguments: src (source file) and dest (destination file).
def file_copy(src, dest):
# Use the 'with' statement to open the source file for reading ('r') and the destination file for writing ('w').
with open(src) as f, open(dest, 'w') as d:
# Read the content of the source file and write it to the destination file.
# Call the file_copy function with the source file "untitled0.py" and the destination file "z.py".
file_copy("untitled0.py", "z.py")
# Use the 'with' statement to open the 'z.py' file for reading ('r').
with open('z.py', 'r') as filehandle:
# Iterate through the lines in the 'z.py' file.
for line in filehandle:
# Print each line, and specify 'end' as an empty string to avoid extra line breaks.
print(line, end = '')
Flowchart:
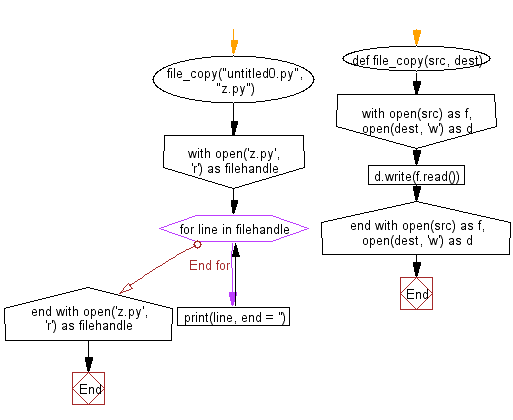
Python Code Editor:
Previous: Write a Python program to perform an action if a condition is true.
Next: Write a Python program to swap two variables.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-basic-exercise-90.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics