Python Exercise: Find numbers which are divisible by 7 and multiple of 5 between a range
1. Divisible by 7 and Multiples of 5
Write a Python program to find those numbers which are divisible by 7 and multiples of 5, between 1500 and 2700 (both included).
Pictorial Presentation:
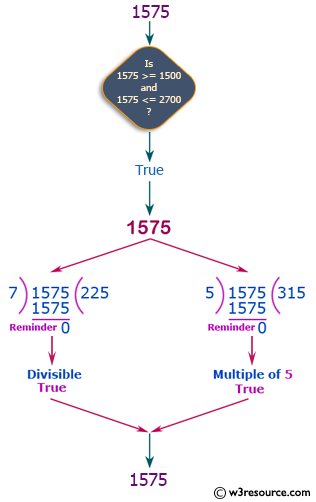
Sample Solution:
Python Code:
# Create an empty list to store numbers that meet the given conditions
nl = []
# Iterate through numbers from 1500 to 2700 (inclusive)
for x in range(1500, 2701):
# Check if the number is divisible by 7 and 5 without any remainder
if (x % 7 == 0) and (x % 5 == 0):
# If the conditions are met, convert the number to a string and append it to the list
nl.append(str(x))
# Join the numbers in the list with a comma and print the result
print(','.join(nl))
Sample Output:
1505,1540,1575,1610,1645,1680,1715,1750,1785,1820,1855,1890,1925,1960,1995,2030,2065,2100,2135,2170,2205,2240, 2275,2310,2345,2380,2415,2450,2485,2520,2555,2590,2625,2660,2695
Flowchart:
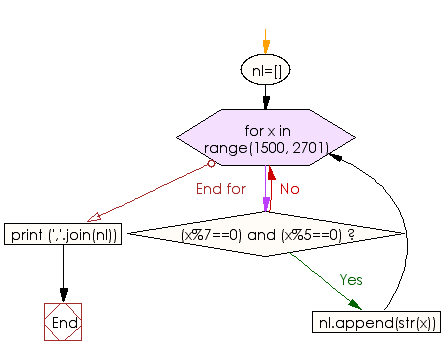
For more Practice: Solve these Related Problems:
- Write a Python program to list numbers between 1000 and 3000 that are divisible by 7 but not by 5.
- Write a Python program to count how many numbers between 1500 and 2700 are divisible by 7 and also multiples of 5.
- Write a Python program to compute the sum of numbers between 1500 and 2700 that are divisible by 7 and multiples of 5.
- Write a Python program to generate a comma-separated string of numbers between 1500 and 2700 that satisfy being divisible by 7 and multiples of 5.
Go to:
Previous: Python Conditional Statements and Loops Exercises Home
Next: Write a Python program to convert temperatures to and from celsius, fahrenheit.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.