Python Exercise: Sequence of lines as input and prints the lines as output in lower case
12. Sequence of Lines to Lowercase
Write a Python program that accepts a sequence of lines (blank line to terminate) as input and prints the lines as output (all characters in lower case).
Pictorial Presentation:
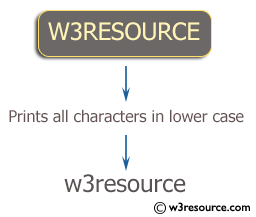
Sample Solution:
Python Code:
# Create an empty list named 'lines'
lines = []
# Continue to prompt the user for input indefinitely until a blank line is entered
while True:
# Prompt the user to input a line of text
l = input()
# Check if the entered line is not empty (non-empty string)
if l:
# Convert the entered line to uppercase and append it to the 'lines' list
lines.append(l.upper())
else:
# If the entered line is empty, break out of the loop
break;
# Iterate through each line in the 'lines' list
for l in lines:
# Print each line (converted to uppercase) from the 'lines' list
print(l)
Sample Output:
w3resource Python W3RESOURCE PYTHON
Flowchart:
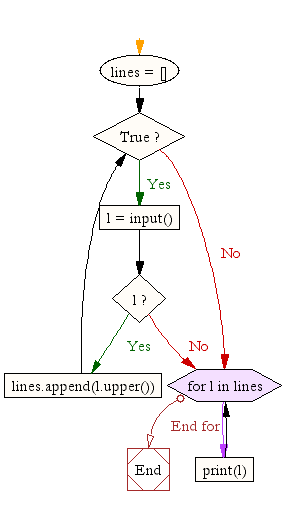
For more Practice: Solve these Related Problems:
- Write a Python program to continuously accept user input lines until a blank line is encountered, then output all lines in lower case.
- Write a Python program to read multiple lines from standard input and convert each line to lower case before printing.
- Write a Python program to build a list of input lines, convert them to lower case, and print the list.
- Write a Python program to implement a loop that terminates on an empty line and prints all captured lines in lower case.
Go to:
Previous: Write a Python program which takes two digits m (row) and n (column) as input and generates a two-dimensional array. The element value in the i-th row and j-th column of the array should be i*j.
Next: Write a Python program which accepts a sequence of comma separated 4 digit binary numbers as its input and print the numbers that are divisible by 5 in a comma separated sequence.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.