Python Exercise: Calculate the number of digits and letters in a string
Python Conditional: Exercise-14 with Solution
Write a Python program that accepts a string and calculates the number of digits and letters.
Pictorial Presentation:
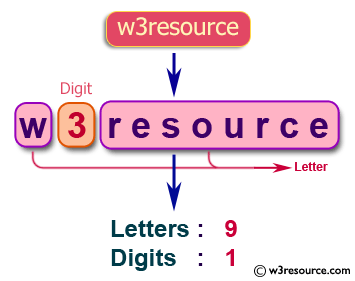
Sample Solution:
Python Code:
# Prompt the user to input a string and store it in the variable 's'
s = input("Input a string")
# Initialize variables 'd' (for counting digits) and 'l' (for counting letters) with values 0
d = l = 0
# Iterate through each character 'c' in the input string 's'
for c in s:
# Check if the current character 'c' is a digit
if c.isdigit():
# If 'c' is a digit, increment the count of digits ('d')
d = d + 1
# Check if the current character 'c' is an alphabet letter
elif c.isalpha():
# If 'c' is an alphabet letter, increment the count of letters ('l')
l = l + 1
else:
# If 'c' is neither a digit nor an alphabet letter, do nothing ('pass')
pass
# Print the total count of letters ('l') and digits ('d') in the input string 's'
print("Letters", l)
print("Digits", d)
Sample Output:
Input a string W3resource Letters 9 Digits 1
Flowchart:
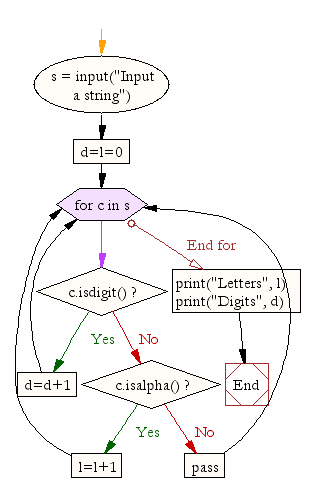
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program which accepts a sequence of comma separated 4 digit binary numbers as its input and print the numbers that are divisible by 5 in a comma separated sequence.
Next: Write a Python program to check the validity of a password (input from users).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-conditional-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics