Python Exercise: Check the validity of a password
Write a Python program to check the validity of passwords input by users.
Validation :
- At least 1 letter between [a-z] and 1 letter between [A-Z].
- At least 1 number between [0-9].
- At least 1 character from [$#@].
- Minimum length 6 characters.
- Maximum length 16 characters.
Sample Solution:-
Python Code:
# Import the 're' module for regular expressions
import re
# Prompt the user to input a password and store it in the variable 'p'
p = input("Input your password")
# Set 'x' to True to enter the while loop
x = True
# Start a while loop that continues until 'x' is True
while x:
# Check conditions for a valid password:
# Password length should be between 6 and 12 characters
if (len(p) < 6 or len(p) > 12):
break
# Password should contain at least one lowercase letter
elif not re.search("[a-z]", p):
break
# Password should contain at least one digit
elif not re.search("[0-9]", p):
break
# Password should contain at least one uppercase letter
elif not re.search("[A-Z]", p):
break
# Password should contain at least one special character among '$', '#', '@'
elif not re.search("[$#@]", p):
break
# Password should not contain any whitespace character
elif re.search("\s", p):
break
else:
# If all conditions are met, print "Valid Password" and set 'x' to False to exit the loop
print("Valid Password")
x = False
break
# If 'x' remains True, print "Not a Valid Password"
if x:
print("Not a Valid Password")
Sample Output:
Input your passwordW3r@100a Valid Password
Flowchart:
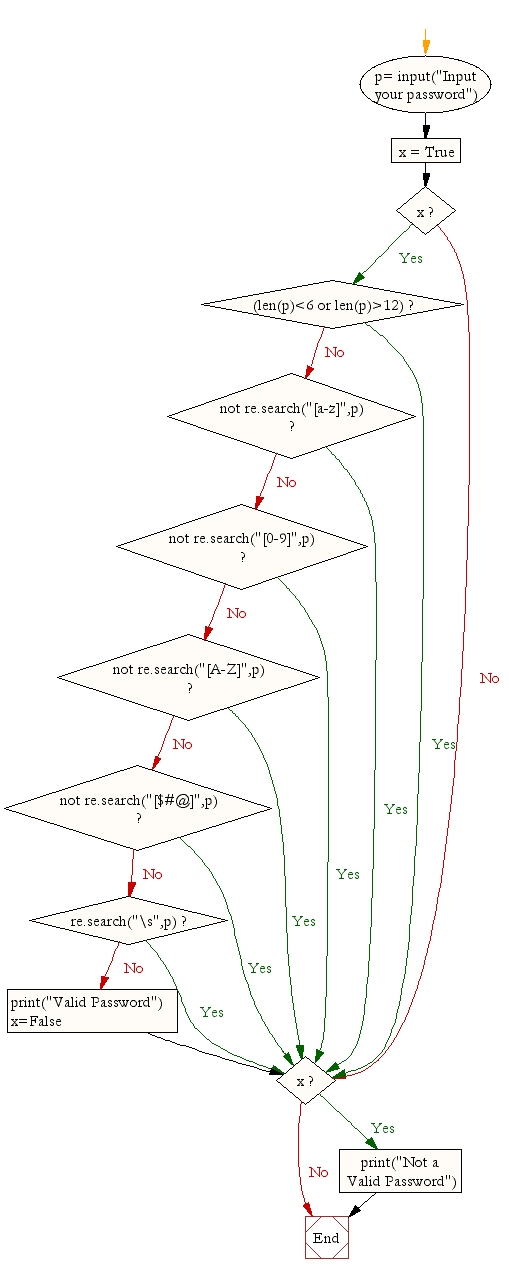
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program that accepts a string and calculate the number of digits and letters.
Next: Write a Python program to find numbers between 100 and 400 (both included) where each digit of a number is an even number. The numbers obtained should be printed in a comma-separated sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics