Python Exercise: Find numbers between two numbers where each digit of a number is an even number
16. Numbers with All Even Digits
Write a Python program to find numbers between 100 and 400 (both included) where each digit of a number is an even number. The numbers obtained should be printed in a comma-separated sequence.
Pictorial Presentation:
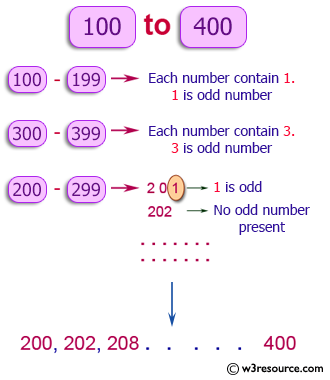
Sample Solution:
Python Code:
# Create an empty list named 'items'
items = []
# Iterate through numbers from 100 to 400 (inclusive) using the range function
for i in range(100, 401):
# Convert the current number 'i' to a string and store it in the variable 's'
s = str(i)
# Check if each digit in the current number 'i' is even (divisible by 2)
if (int(s[0]) % 2 == 0) and (int(s[1]) % 2 == 0) and (int(s[2]) % 2 == 0):
# If all digits are even, append the string representation of 'i' to the 'items' list
items.append(s)
# Join the elements in the 'items' list separated by ',' and print the result
print(",".join(items))
Sample Output:
200,202,204,206,208,220,222,224,226,228,240,242,244,246,248,260,262,264,266,268,280,282,284,286,288,400
Flowchart:
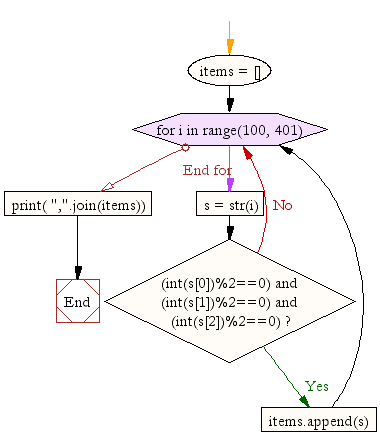
For more Practice: Solve these Related Problems:
- Write a Python program to list all numbers between 100 and 400 whose every digit is even.
- Write a Python program to filter numbers in a range by converting them to strings and checking if each digit is even.
- Write a Python program to use list comprehension to output a comma-separated string of numbers with only even digits.
- Write a Python program to implement a function that tests whether all digits in a number are even and apply it to a specified range.
Go to:
Previous: Write a Python program to check the validity of a password (input from users).
Next: Write a Python program to print alphabet pattern 'A'.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.