Python Exercise: Guess a number between 1 to 9
Python Conditional: Exercise-3 with Solution
Write a Python program to guess a number between 1 and 9.
Note : User is prompted to enter a guess. If the user guesses wrong then the prompt appears again until the guess is correct, on successful guess, user will get a "Well guessed!" message, and the program will exit.
Sample Solution:
Python Code:
# Import the 'random' module to generate random numbers
import random
# Generate a random number between 1 and 10 (inclusive) as the target number
target_num, guess_num = random.randint(1, 10), 0
# Start a loop that continues until the guessed number matches the target number
while target_num != guess_num:
# Prompt the user to input a number between 1 and 10 and convert it to an integer
guess_num = int(input('Guess a number between 1 and 10 until you get it right : '))
# Print a message indicating successful guessing once the correct number is guessed
print('Well guessed!')
Sample Output:
Guess a number between 1 and 10 until you get it right : 5 Well guessed!
Flowchart:
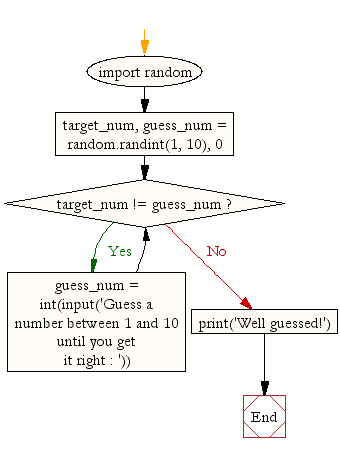
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to convert temperatures to and from celsius, fahrenheit.
Next: Write a Python program to construct the following pattern, using a nested for loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-conditional-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics