Python Exercise: Print alphabet pattern Z
30. Alphabet Pattern 'Z'
Write a Python program to print the alphabet pattern 'Z'.
Pictorial Presentation:
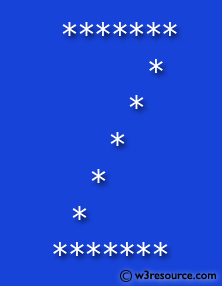
Sample Solution:
Python Code:
# Initialize an empty string named 'result_str'
result_str = ""
# Loop through rows from 0 to 6 using the range function
for row in range(0, 7):
# Loop through columns from 0 to 6 using the range function
for column in range(0, 7):
# Check conditions to determine whether to place '*' or ' ' in the result string
# If conditions are met, place '*' in specific positions based on row and column values
if (((row == 0 or row == 6) and column >= 0 and column <= 6) or row + column == 6):
result_str = result_str + "*" # Append '*' to the 'result_str'
else:
result_str = result_str + " " # Append space (' ') to the 'result_str'
result_str = result_str + "\n" # Add a newline character after each row in 'result_str'
# Print the final 'result_str' containing the pattern
print(result_str)
Sample Output:
******* * * * * * *******
Flowchart :
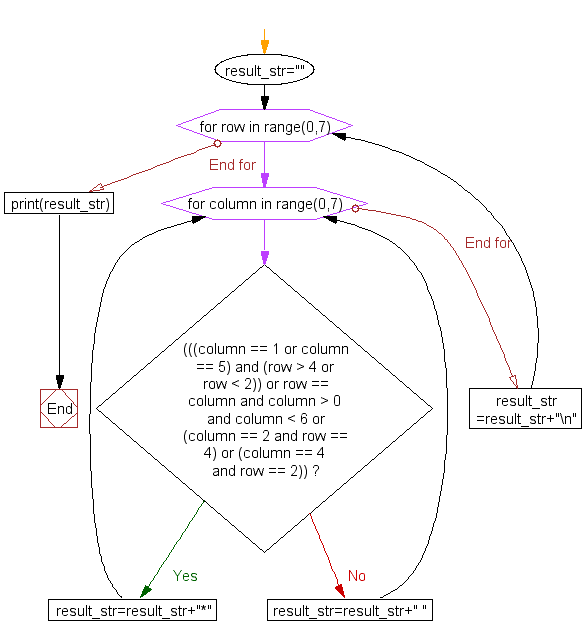
For more Practice: Solve these Related Problems:
- Write a Python program to print the letter 'Z' pattern with a full top and bottom line and a diagonal connecting them.
- Write a Python program to generate 'Z' using loops, ensuring the diagonal shifts one position per row.
- Write a Python program to construct the pattern for 'Z' by calculating the required number of spaces for the diagonal.
- Write a Python program to implement 'Z' using string concatenation and a loop to insert the diagonal correctly.
Go to:
Previous: Write a Python program to print alphabet pattern 'X'.
Next: Write a Python program to calculate a dog's age in dog's years.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.