Python Exercise: Check whether an alphabet is a vowel or consonant
32. Vowel or Consonant Checker
Write a Python program to check whether an alphabet is a vowel or consonant.
Pictorial Presentation:
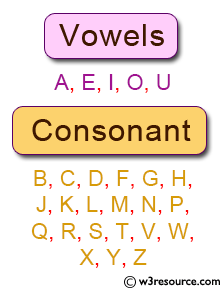
Sample Solution:
Python Code:
# Request input from the user to input a letter of the alphabet and assign it to the variable 'l'
l = input("Input a letter of the alphabet: ")
# Check if the input letter 'l' is present in the tuple containing vowels ('a', 'e', 'i', 'o', 'u')
if l in ('a', 'e', 'i', 'o', 'u'):
print("%s is a vowel." % l) # Display a message stating that the input letter is a vowel
# If the input letter is 'y', display a message stating that it sometimes represents a vowel and sometimes a consonant
elif l == 'y':
print("Sometimes the letter y stands for a vowel, sometimes for a consonant.")
# If the input letter doesn't fall into any of the above conditions, it is considered a consonant; display a message stating so
else:
print("%s is a consonant." % l)
Sample Output:
Input a letter of the alphabet: u u is a vowel.
Flowchart:
Python Code Editor:
For more Practice: Solve these Related Problems:
- Write a Python program to check if a given letter is a vowel or a consonant, using a set of vowels for comparison.
- Write a Python program to accept a letter and determine its classification, ensuring input validation for alphabets only.
- Write a Python program to implement a function that returns "vowel" or "consonant" for a single-character input.
- Write a Python program to handle both uppercase and lowercase input and output the correct classification.
Go to:
Previous: Write a Python program to calculate a dog's age in dog's years.
Next: Write a Python program to convert month name to a number of days.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.