Python Exercise: Prints the season for that month and day
37. Season Finder from Date
Write a Python program that reads two integers representing a month and day and prints the season for that month and day.
Pictorial Presentation:
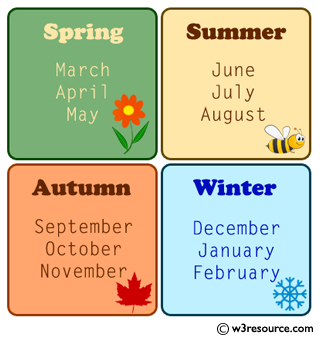
Sample Solution:
Python Code:
# Request input from the user for the name of the month and assign it to the variable 'month'
month = input("Input the month (e.g. January, February etc.): ")
# Request input from the user for the day and convert it to an integer, assigning it to the variable 'day'
day = int(input("Input the day: "))
# Check the input 'month' to determine the season based on the month and day provided
# Check if the input month falls within the winter season
if month in ('January', 'February', 'March'):
season = 'winter'
# Check if the input month falls within the spring season
elif month in ('April', 'May', 'June'):
season = 'spring'
# Check if the input month falls within the summer season
elif month in ('July', 'August', 'September'):
season = 'summer'
# For other months, assign the season as autumn by default
else:
season = 'autumn'
# Adjust the season based on the specific day within certain months
# Check if the input month is March and the day is after March 19, updating the season to spring
if (month == 'March') and (day > 19):
season = 'spring'
# Check if the input month is June and the day is after June 20, updating the season to summer
elif (month == 'June') and (day > 20):
season = 'summer'
# Check if the input month is September and the day is after September 21, updating the season to autumn
elif (month == 'September') and (day > 21):
season = 'autumn'
# Check if the input month is December and the day is after December 20, updating the season to winter
elif (month == 'December') and (day > 20):
season = 'winter'
# Display the determined season based on the provided month and day
print("Season is", season)
Sample Output:
Input the month (e.g. January, February etc.): April Input the day: 15 Season is spring
Flowchart :
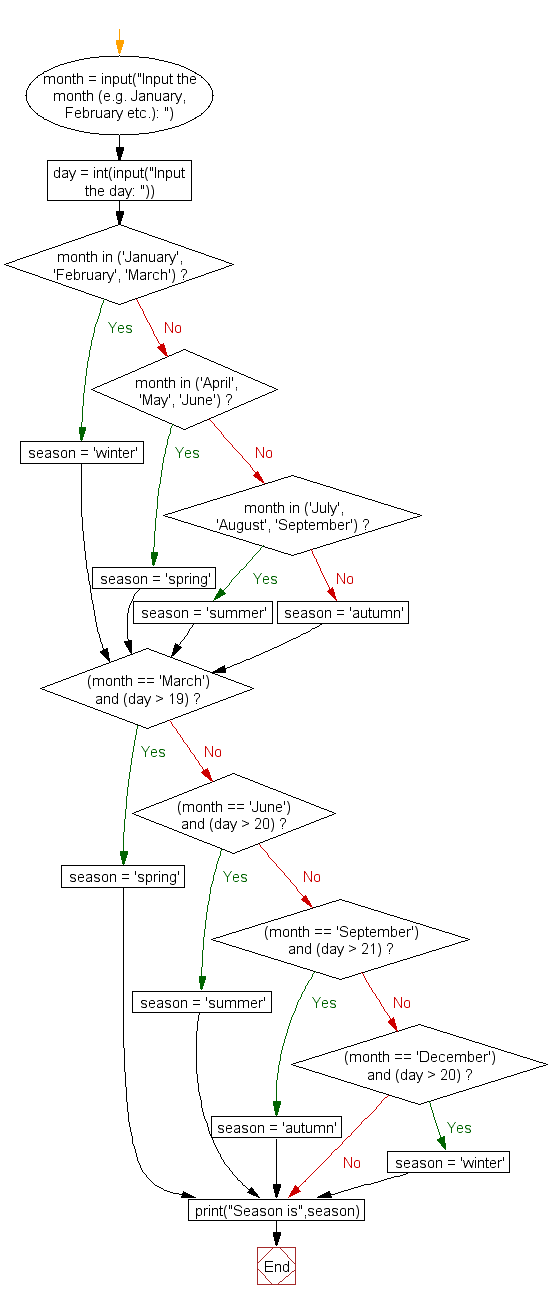
For more Practice: Solve these Related Problems:
- Write a Python program to determine the season of the year given a month and day by mapping dates to seasons.
- Write a Python program to use a dictionary or if-else ladder to output the season based on user input for month and day.
- Write a Python program to check boundary dates and return the appropriate season for a given month-day combination.
- Write a Python program to implement a function that takes a month and day and returns "spring", "summer", "autumn", or "winter".
Go to:
Previous: Write a Python program to check a triangle is equilateral, isosceles or scalene.
Next: Write a Python program to display astrological sign for given date of birth.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.