Python Exercise: Display sign of the Chinese Zodiac for given year
39. Chinese Zodiac Sign
Write a Python program to display the sign of the Chinese Zodiac for the given year in which you were born.
Sample Solution:
Python Code:
# Request input from the user for the birth year and convert it to an integer, assigning it to the variable 'year'
year = int(input("Input your birth year: "))
# Determine the Chinese zodiac sign based on the provided birth year
# Check if the year corresponds to the Chinese zodiac sign 'Dragon'
if (year - 2000) % 12 == 0:
sign = 'Dragon'
# Check if the year corresponds to the Chinese zodiac sign 'Snake'
elif (year - 2000) % 12 == 1:
sign = 'Snake'
# Check if the year corresponds to the Chinese zodiac sign 'Horse'
elif (year - 2000) % 12 == 2:
sign = 'Horse'
# Check if the year corresponds to the Chinese zodiac sign 'Sheep'
elif (year - 2000) % 12 == 3:
sign = 'Sheep'
# Check if the year corresponds to the Chinese zodiac sign 'Monkey'
elif (year - 2000) % 12 == 4:
sign = 'Monkey'
# Check if the year corresponds to the Chinese zodiac sign 'Rooster'
elif (year - 2000) % 12 == 5:
sign = 'Rooster'
# Check if the year corresponds to the Chinese zodiac sign 'Dog'
elif (year - 2000) % 12 == 6:
sign = 'Dog'
# Check if the year corresponds to the Chinese zodiac sign 'Pig'
elif (year - 2000) % 12 == 7:
sign = 'Pig'
# Check if the year corresponds to the Chinese zodiac sign 'Rat'
elif (year - 2000) % 12 == 8:
sign = 'Rat'
# Check if the year corresponds to the Chinese zodiac sign 'Ox'
elif (year - 2000) % 12 == 9:
sign = 'Ox'
# Check if the year corresponds to the Chinese zodiac sign 'Tiger'
elif (year - 2000) % 12 == 10:
sign = 'Tiger'
# If the year doesn't correspond to any specific sign, assign it to 'Hare'
else:
sign = 'Hare'
# Display the determined Chinese zodiac sign based on the provided birth year
print("Your Zodiac sign :", sign)
Sample Output:
Input your birth year: 1784 Your Zodiac sign : Dragon
Flowchart :
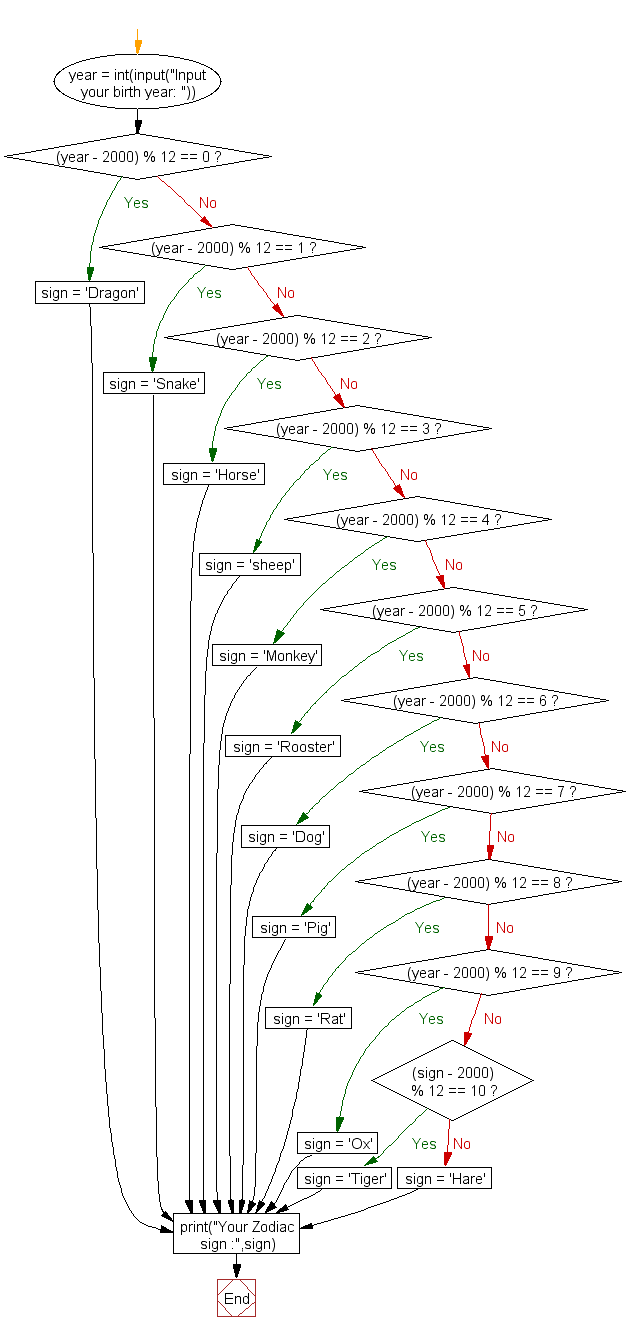
For more Practice: Solve these Related Problems:
- Write a Python program to determine the Chinese Zodiac sign for a given year by calculating the remainder when divided by 12.
- Write a Python program to use a list of zodiac animals and map a given birth year to its corresponding sign.
- Write a Python program to prompt the user for a birth year and output the associated Chinese Zodiac animal.
- Write a Python program to implement a function that returns the Zodiac sign from a year using modular arithmetic.
Go to:
Previous: Write a Python program to display astrological sign for given date of birth.
Next: Write a Python program to find the median of three values.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.