Handling AttributeError Exception in Python list operation program
10. Handle AttributeError Exception in List Operations
Write a Python program that executes a list operation and handles an AttributeError exception if the attribute does not exist.
exception AttributeError:
Raised when an attribute reference (see Attribute references) or assignment fails. (When an object does not support attribute references or attribute assignments at all, TypeError is raised.)
Sample Solution:
Code:
# Define a function named 'test_list_operation' that takes 'nums' as a parameter.
def test_list_operation(nums):
try:
# Attempt to access the 'length' attribute of the 'nums' list and assign it to 'r'.
r = len(nums) # Trying to access the length attribute
# Print the length of the list 'nums'.
print("Length of the list:", r)
except AttributeError:
# Handle the exception if an AttributeError occurs when attempting to access the 'length' attribute.
print("Error: The list does not have a 'length' attribute.")
# Create a list 'nums' containing integer values.
nums = [1, 2, 3, 4, 5]
# Call the 'test_list_operation' function with the 'nums' list as a parameter to check for the 'length' attribute.
test_list_operation(nums)
Output:
Length of the list: 5
Flowchart:
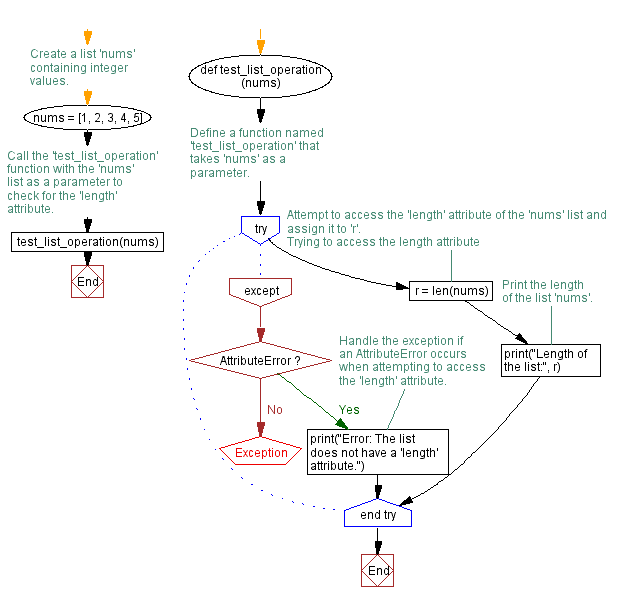
Code:
# Define a function named 'test_list_operation' that takes 'nums' as a parameter.
def test_list_operation(nums):
try:
# Attempt to access an undefined attribute 'lengtha' of the 'nums' list and assign it to 'r1'.
r1 = nums.lengtha # Trying to access the 'length' attribute
# Print the result, which will not execute due to the exception.
print("Length of the list:", r1)
except AttributeError:
# Handle the exception if an AttributeError occurs when attempting to access the 'lengtha' attribute.
print("Error: The list does not have a 'lengtha' attribute.")
# Create a list 'nums' containing integer values.
nums = [1, 2, 3, 4, 5]
# Call the 'test_list_operation' function with the 'nums' list as a parameter to check for the 'lengtha' attribute.
test_list_operation(nums)
Explanation:
In the above exercise,
- The "test_list_operation() function is defined, which takes a list, "nums", as a parameter.
- Inside the function, we use a try block to perform the list operation. In this case, we try to access the list length using the len() function and store it in the length variable.
- If the len() function is successfully executed, we print the list length.
- However, if an AttributeError exception occurs because the list does not have a length attribute, the program jumps to the except block. In this block, we catch the AttributeError exception and print an error message indicating that the list does not have a length attribute.
Output:
Error: The list does not have a 'length' attribute.
Flowchart:
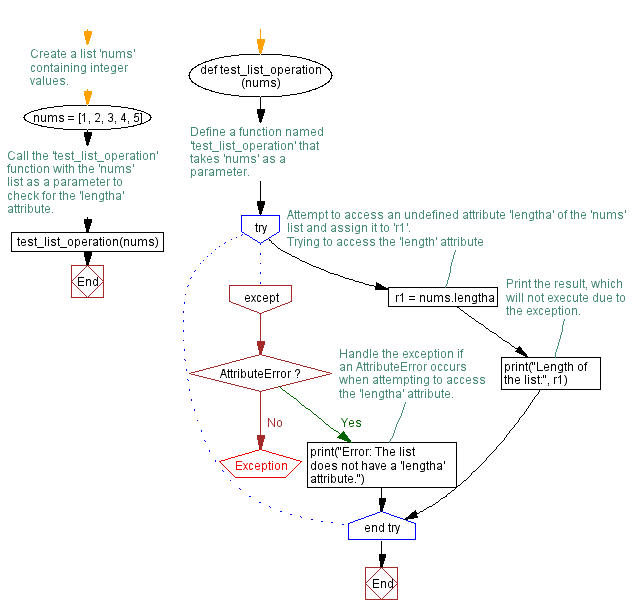
For more Practice: Solve these Related Problems:
- Write a Python program to attempt to access a non-existent attribute of a list and catch the resulting AttributeError.
- Write a Python program to implement a function that tests if a list has a specific method and raises an AttributeError if it doesn't.
- Write a Python program to simulate an AttributeError by mistakenly calling a method on a non-list object, then handling the exception.
- Write a Python program to use try-except to verify that a given list supports a particular attribute before performing an operation.
Go to:
Previous: Handling UnicodeDecodeError Exception in Python file Handling program.
Next: Python Object-Oriented Program Home
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.