Python Exercise: Detect the number of local variables declared in a function
20. Detect the Number of Local Variables Declared in a Function
Write a Python program to detect the number of local variables declared in a function.
Sample Solution:
Python Code:
# Define a function named 'abc'
def abc():
# Define and assign values to local variables 'x', 'y', and 'str1' inside the function 'abc'
x = 1
y = 2
str1 = "w3resource"
# Print the string "Python Exercises"
print("Python Exercises")
# Access the number of local variables in the function 'abc' using the __code__.co_nlocals attribute
print(abc.__code__.co_nlocals)
Sample Output:
3
Explanation:
In the exercise above the code defines a function "abc()" that contains three local variables: 'x', 'y', and 'str1'. However, it only defines these variables and doesn't utilize them further. Then, it accesses the number of local variables defined in the "abc()" function using the abc.__code__.co_nlocals attribute and prints the result.
Flowchart:
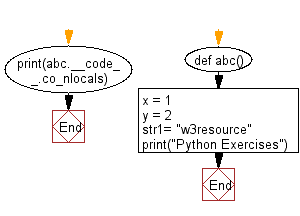
For more Practice: Solve these Related Problems:
- Write a Python program that defines a function with multiple local variables and then prints the count using len(locals()).
- Write a Python program to create a function that returns the list of all local variable names using the __code__.co_varnames attribute.
- Write a Python program to compare the number of local variables between two functions using the inspect module.
- Write a Python program to implement a function that dynamically adds local variables and then returns the total count using locals().
Go to:
Previous: Write a Python program to execute a string containing Python code.
Next: Write a Python program that invoke a given function after specific milliseconds.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.