Python Exercise: Reverse a string
4. Reverse a String
Write a Python program to reverse a string.
Sample String: "1234abcd"
Expected Output: "dcba4321"
Sample Solution-1:
Python Code:
# Define a function named 'string_reverse' that takes a string 'str1' as input
def string_reverse(str1):
# Initialize an empty string 'rstr1' to store the reversed string
rstr1 = ''
# Calculate the length of the input string 'str1'
index = len(str1)
# Execute a while loop until 'index' becomes 0
while index > 0:
# Concatenate the character at index - 1 of 'str1' to 'rstr1'
rstr1 += str1[index - 1]
# Decrement the 'index' by 1 for the next iteration
index = index - 1
# Return the reversed string stored in 'rstr1'
return rstr1
# Print the result of calling the 'string_reverse' function with the input string '1234abcd'
print(string_reverse('1234abcd'))
Sample Output:
dcba4321
Explanation:
In the exercise above the code defines a function named "string_reverse()" that takes a string as input and returns its reversed version using a while loop and string manipulation. The final print statement demonstrates the result of calling the "string_reverse()" function with the input string '1234abcd'.
Pictorial presentation:
Flowchart:
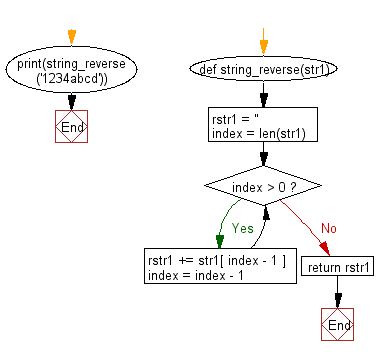
Sample Solution-2:
Python Code:
# Define a function named 'reverse' that takes an iterable 'itr' as input and returns its reverse
def reverse(itr):
# Using slicing with [::-1] reverses the input 'itr' and returns the reversed version
return itr[::-1]
# Initialize a string variable 'str1' with the value '1234abcd'
str1 = '1234abcd'
# Print the original string 'str1'
print("Original string:", str1)
# Print the reversed string obtained by calling the 'reverse' function with the string '1234abcd'
print("Reverse string:", reverse('1234abcd'))
# Change the value of 'str1' to 'reverse'
str1 = 'reverse'
# Print the original string 'str1'
print("\nOriginal string:", str1)
# Print the reversed string obtained by calling the 'reverse' function with the string 'reverse'
print("Reverse string:", reverse(str1))
Sample Output:
Original string: 1234abcd Reverse strig: dcba4321 Original string: reverse Reverse string: esrever
Explanation:
In the exercise above the code demonstrates the use of a "reverse()" function that utilizes slicing ([::-1]) to reverse a given input iterable. It then applies this function to reverse both the string '1234abcd' and the string 'reverse' and prints their original and reversed versions.
Flowchart:
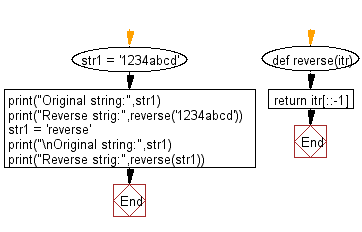
For more Practice: Solve these Related Problems:
- Write a Python function that uses slicing to reverse a given string.
- Write a Python function that iterates over the string in reverse order using a loop and constructs the reversed string.
- Write a Python function that converts the string to a list, reverses the list, and joins it back into a string.
- Write a Python function that uses recursion to reverse a string and returns the result.
Go to:
Previous: Write a Python function to multiply all the numbers in a list.
Next: Write a Python function to calculate the factorial of a number (a non-negative integer). The function accepts the number as an argument.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.