Python JSON: Check whether a JSON string contains complex object or not
Python JSON: Exercise-8 with Solution
Write a Python program to check whether a JSON string contains complex object or not.
Sample Solution:-
Python Code:
import json
def is_complex_num(objct):
if '__complex__' in objct:
return complex(objct['real'], objct['img'])
return objct
complex_object =json.loads('{"__complex__": true, "real": 4, "img": 5}', object_hook = is_complex_num)
simple_object =json.loads('{"real": 4, "img": 3}', object_hook = is_complex_num)
print("Complex_object: ",complex_object)
print("Without complex object: ",simple_object)
Output:
Complex_object: (4+5j) Without complex object: {'real': 4, 'img': 3}
Flowchart:
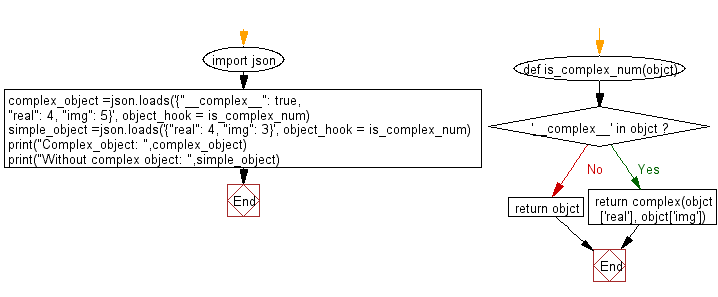
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check whether an instance is complex or not.
Next: Write a Python program to access only unique key value of a Python object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/python-json-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics