Python: Search some literals strings in a string
Python Regular Expression: Exercise-19 with Solution
Write a Python program to search for literal strings within a string.
Sample Solution:
Python Code:
import re
patterns = [ 'fox', 'dog', 'horse' ]
text = 'The quick brown fox jumps over the lazy dog.'
for pattern in patterns:
print('Searching for "%s" in "%s" ->' % (pattern, text),)
if re.search(pattern, text):
print('Matched!')
else:
print('Not Matched!')
Sample Output:
Searching for "fox" in "The quick brown fox jumps over the lazy dog." -> Matched! Searching for "dog" in "The quick brown fox jumps over the lazy dog." -> Matched! Searching for "horse" in "The quick brown fox jumps over the lazy dog." -> Not Matched!
Pictorial Presentation:
Flowchart:
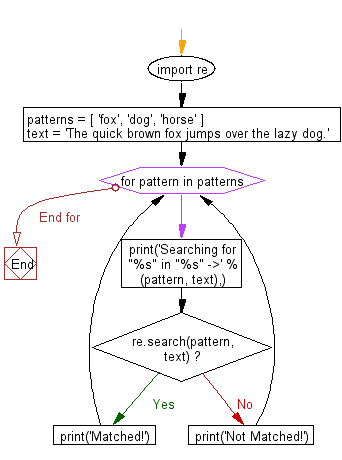
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write Python program to search the numbers (0-9) of length between 1 to 3 in a given string.
Next: Write a Python program to search a literals string in a string and also find the location within the original string where the pattern occurs.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/re/python-re-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics