Python Exercises: Two following words begin and end with a vowel
58. Consecutive Vowel Check
From Wikipedia,
A vowel is a syllabic speech sound pronounced without any stricture in the vocal tract. Vowels are one of the two principal classes of speech sounds, the other being the consonant.
Write a Python program that takes a string with some words. For two consecutive words in the said string, check whether the first word ends with a vowel and the next word begins with a vowel. If the program meets the condition, return true, otherwise false. Only one space is allowed between the words.
Sample Data:
("These exercises can be used for practice.") -> True
("Following exercises should be removed for practice.") -> False
("I use these stories in my classroom.") -> True
Sample Solution-1:
Python Code:
import re
def test(text):
return bool(re.findall('[AEIOUaeiou] [AEIOUaeiou]', text))
text ="These exercises can be used for practice."
print("Original string:", text)
print("Two following words begin and end with a vowel in the said string:")
print(test(text))
text ="Following exercises should be removed for practice."
print("\nOriginal string:", text)
print("Two following words begin and end with a vowel in the said string:")
print(test(text))
text ="I use these stories in my classroom."
print("\nOriginal string:", text)
print("Two following words begin and end with a vowel in the said string:")
print(test(text))
Sample Output:
Original string: These exercises can be used for practice. Two following words begin and end with a vowel in the said string: True Original string: Following exercises should be removed for practice. Two following words begin and end with a vowel in the said string: False Original string: I use these stories in my classroom. Two following words begin and end with a vowel in the said string: True
Flowchart:
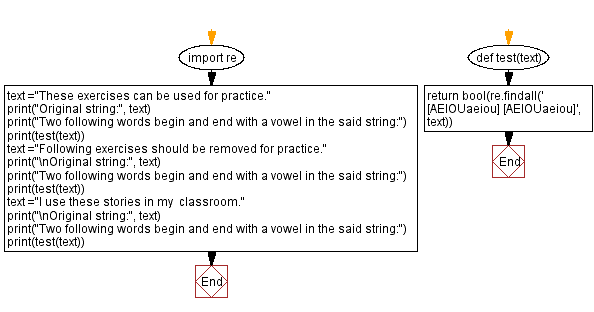
Sample Solution-2:
Python Code:
import re
def test(text):
result_regex = re.compile(r"[AEIOUaeiou]\s[AEIOUaeiou]")
if (result_regex.search(text)):
return True
return False
text ="These exercises can be used for practice."
print("Original string:", text)
print("Two following words begin and end with a vowel in the said string:")
print(test(text))
text ="Following exercises should be removed for practice."
print("\nOriginal string:", text)
print("Two following words begin and end with a vowel in the said string:")
print(test(text))
text ="I use these stories in my classroom."
print("\nOriginal string:", text)
print("Two following words begin and end with a vowel in the said string:")
print(test(text))
Sample Output:
Original string: These exercises can be used for practice. Two following words begin and end with a vowel in the said string: True Original string: Following exercises should be removed for practice. Two following words begin and end with a vowel in the said string: False Original string: I use these stories in my classroom. Two following words begin and end with a vowel in the said string: True
Flowchart:
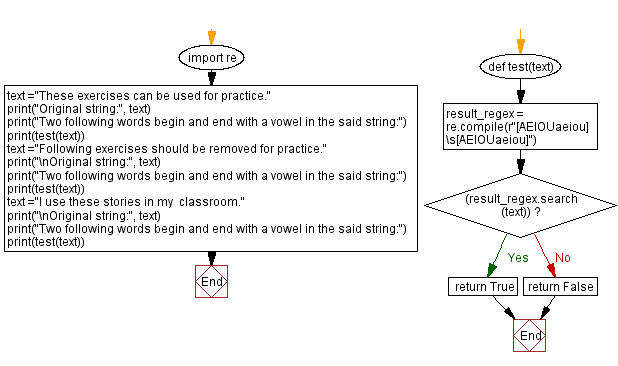
For more Practice: Solve these Related Problems:
- Write a Python program to check a string for pairs of consecutive words where the first ends with a vowel and the second begins with a vowel.
- Write a Python script to analyze a sentence and return True if any adjacent word pair meets the vowel-ending and vowel-starting condition.
- Write a Python program to scan a string with multiple words and determine if at least one pair of consecutive words has the required vowel pattern.
- Write a Python function that takes a sentence and verifies whether there is any instance where a word ending in a vowel is immediately followed by a word starting with a vowel.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous Python Exercise: Convert a given string to snake case.
Next: Python NumPy Array Object Exercises Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.