Python: Find sequences of lowercase letters joined with a underscore
7. Lowercase with Underscore
Write a Python program to find sequences of lowercase letters joined by an underscore.
Sample Solution:
Python Code:
import re
def text_match(text):
patterns = '^[a-z]+_[a-z]+$'
if re.search(patterns, text):
return 'Found a match!'
else:
return('Not matched!')
print(text_match("aab_cbbbc"))
print(text_match("aab_Abbbc"))
print(text_match("Aaab_abbbc"))
Sample Output:
Found a match! Not matched! Not matched!
Pictorial Presentation:
Flowchart:
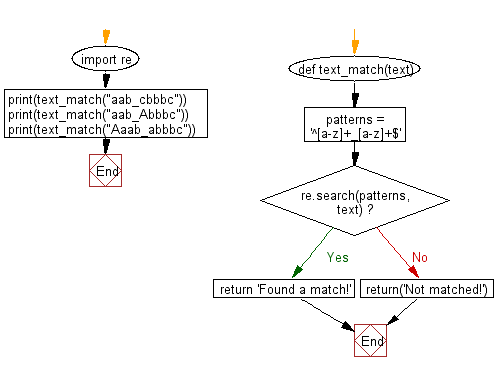
For more Practice: Solve these Related Problems:
- Write a Python program to find all sequences of lowercase letters joined by an underscore in a text.
- Write a Python script to extract and list all substrings that match the pattern of lowercase letters separated by an underscore.
- Write a Python program to identify and count all underscore-connected lowercase word sequences in a given string.
- Write a Python program to replace underscores with spaces in all sequences of lowercase letters found in a text.
Go to:
Previous: Write a Python program that matches a string that has an a followed by two to three 'b'.
Next: Write a Python program to find sequences of one upper case letter followed by lower case letters.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.