Python: Check if a given value is present in a set or not
Write a Python program to check if a given value is present in a set or not.
Visual Presentation:
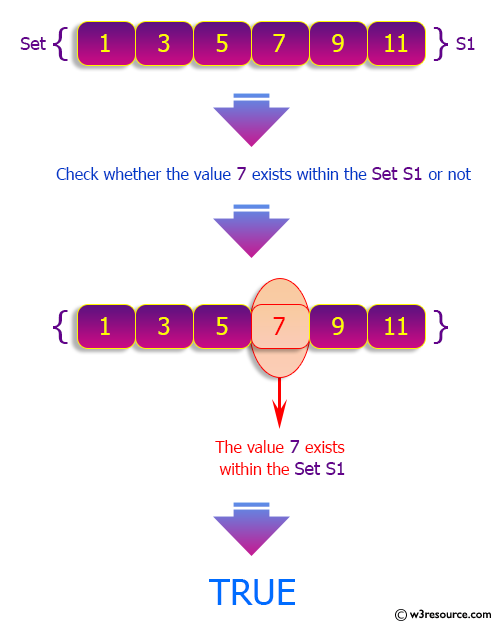
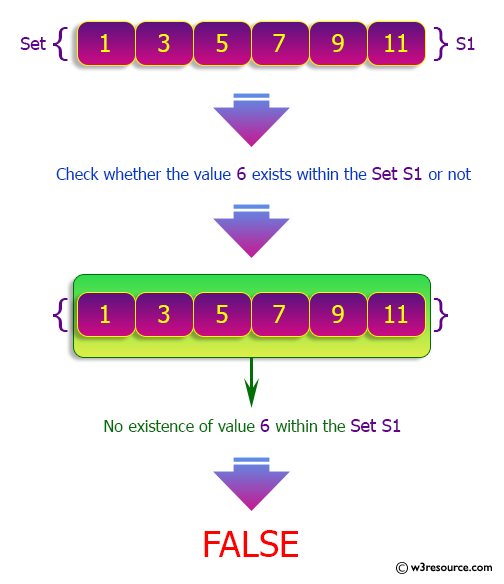
Sample Solution:
Python Code:
# Create a set 'nums' with elements 1, 3, 5, 7, 9, and 11.
nums = {1, 3, 5, 7, 9, 11}
# Print a message to indicate the original set 'nums'.
print("Original set (nums): ", nums, "\n")
# Test if the number 6 exists in 'nums' and print the result.
print("Test if 6 exists in nums:")
print(6 in nums)
# Test if the number 7 exists in 'nums' and print the result.
print("Test if 7 exists in nums:")
print(7 in nums)
# Test if the number 11 exists in 'nums' and print the result.
print("Test if 11 exists in nums:")
print(11 in nums)
# Test if the number 0 exists in 'nums' and print the result.
print("Test if 0 exists in nums:")
print(0 in nums)
# Test if the number 6 is not in 'nums' and print the result.
print("\nTest if 6 is not in nums")
print(6 not in nums)
# Test if the number 7 is not in 'nums' and print the result.
print("Test if 7 is not in nums")
print(7 not in nums)
# Test if the number 11 is not in 'nums' and print the result.
print("Test if 11 is not in nums")
print(11 not in nums)
# Test if the number 0 is not in 'nums' and print the result.
print("Test if 0 is not in nums")
print(0 not in nums)
Sample Output:
Original sets(nums): {1, 3, 5, 7, 9, 11} Test if 6 exists in nums: False Test if 7 exists in nums: True Test if 11 exists in nums: True Test if 0 exists in nums: False Test if 6 is not in nums True Test if 7 is not in nums False Test if 11 is not in nums False Test if 0 is not in nums True
Python Code Editor:
Previous: Write a Python program to find the length of a set.
Next: Write a Python program to check if two given sets have no elements in common.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics