Python: Check if two given sets have no elements in common
Python sets: Exercise-17 with Solution
Write a Python program to check if two given sets have no elements in common.
Visual Presentation:
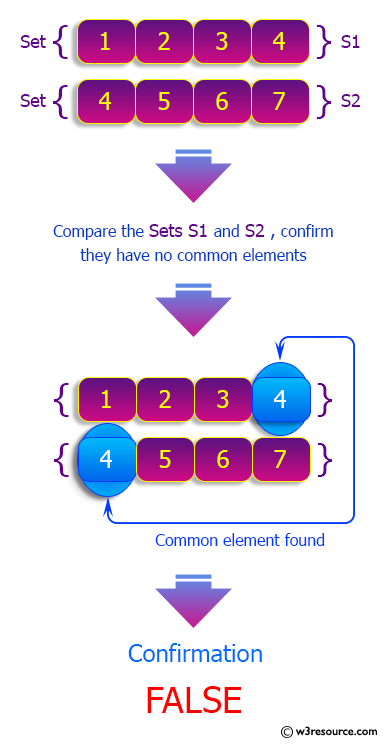
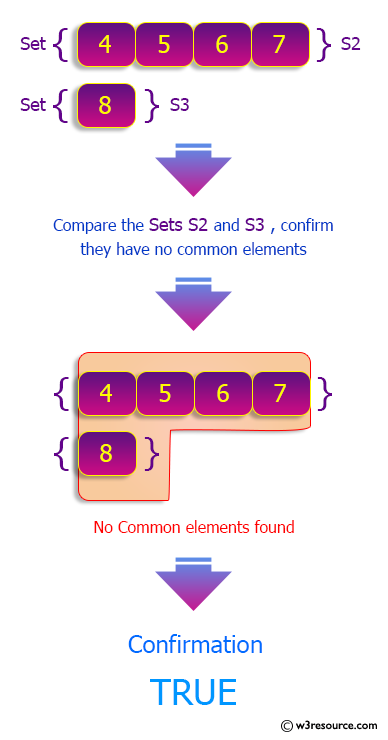
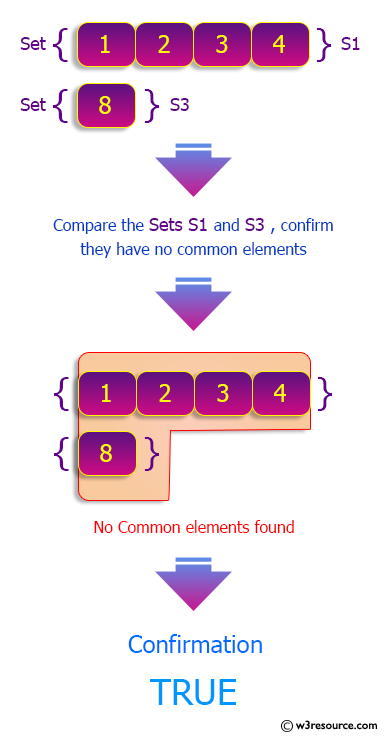
Sample Solution-1:
Python Code:
# Create sets 'x', 'y', and 'z' with different elements.
x = {1, 2, 3, 4}
y = {4, 5, 6, 7}
z = {8}
# Print a message to indicate the original set elements.
print("Original set elements:")
print(x)
print(y)
print(z)
# Print a message to confirm that two given sets have no common elements.
print("\nConfirm two given sets have no element(s) in common:")
# Print a message to indicate comparing sets 'x' and 'y'.
print("\nCompare x and y:")
# Use the 'isdisjoint()' method to check if 'x' and 'y' have no common elements and print the result.
print(x.isdisjoint(y))
# Print a message to indicate comparing sets 'x' and 'z'.
print("\nCompare x and z:")
# Use the 'isdisjoint()' method to check if 'x' and 'z' have no common elements and print the result.
print(z.isdisjoint(x))
# Print a message to indicate comparing sets 'y' and 'z'.
print("\nCompare y and z:")
# Use the 'isdisjoint()' method to check if 'y' and 'z' have no common elements and print the result.
print(y.isdisjoint(z))
Sample Output:
Original set elements: {1, 2, 3, 4} {4, 5, 6, 7} {8} Confirm two given sets have no element(s) in common: Compare x and y: False Compare x and z: True Compare y and z: True
Sample Solution-2:
Python Code:
# Define a function 'compare_sets' to check if two sets have no common elements.
def compare_sets(s1, s2):
for i in s1:
if i in s2:
return False
return True
# Create sets 'x', 'y', and 'z' with different elements.
x = {1, 2, 3, 4}
y = {4, 5, 6, 7}
z = {8}
# Print a message to indicate the original set elements.
print("Original set elements:")
print(x)
print(y)
print(z)
# Print a message to confirm that two given sets have no common elements.
print("\nConfirm two given sets have no element(s) in common:")
# Print a message to indicate comparing sets 'x' and 'y'.
print("\nCompare x and y:")
# Call the 'compare_sets' function to check if 'x' and 'y' have no common elements and print the result.
print(compare_sets(x, y))
# Print a message to indicate comparing sets 'x' and 'z'.
print("\nCompare x and z:")
# Call the 'compare_sets' function to check if 'x' and 'z' have no common elements and print the result.
print(compare_sets(x, z))
# Print a message to indicate comparing sets 'y' and 'z'.
print("\nCompare y and z:")
# Call the 'compare_sets' function to check if 'y' and 'z' have no common elements and print the result.
print(compare_sets(y, z))
Sample Output:
Original set elements: {1, 2, 3, 4} {4, 5, 6, 7} {8} Confirm two given sets have no element(s) in common: Compare x and y: False Compare x and z: True Compare y and z: True
Flowchart:
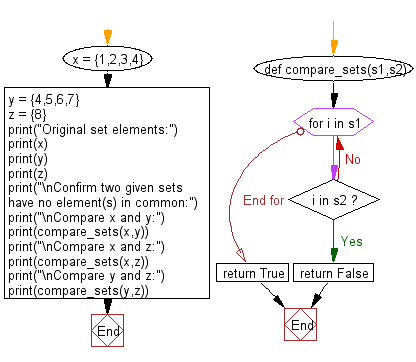
Python Code Editor:
Previous: Write a Python program to check if a given value is present in a set or not.
Next: Write a Python program to check if a given set is superset of itself and superset of another given set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/sets/python-sets-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics