Python: Find all pairs in a list whose sum is equal to a target value
22. Find All Pairs in a List with Sum Equal to a Given Value
Write a Python program that finds all pairs of elements in a list whose sum is equal to a given value.
Sample Solution:
Python Code:
# Define a function 'find_pairs' that takes a list of numbers 'nums' and a target value 'target_val'.
def find_pairs(nums, target_val):
# Create a set 'nums_set' to efficiently check for the presence of numbers in the input list.
nums_set = set(nums)
# Create an empty list 'pairs' to store pairs of numbers that sum up to the target value.
pairs = []
# Iterate over the unique numbers in 'nums_set'.
for n in nums_set:
# Calculate the complement of the current number with respect to the target value.
complement = target_val - n
# Check if the complement is in the 'nums_set'.
if complement in nums_set:
# If the complement exists in the set, add a pair consisting of the current number and its complement to the 'pairs' list.
pairs.append({n, complement})
# Return the 'pairs' list containing pairs of numbers that meet the criteria.
return pairs
nums = [10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
print("Original list of numbers:")
print(nums)
target_val = 35
print("Target value:",target_val)
print("Find all pairs in the said list whose sum is equal to a target value:")
print(find_pairs(nums, target_val))
nums = [1, 2, 3, 4, 5]
print("\nOriginal list of numbers:")
print(nums)
target_val = 5
print("Target value:",target_val)
print("Find all pairs in the said list whose sum is equal to a target value:")
print(find_pairs(nums, target_val))
Sample Output:
Original list of numbers: [10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20] Target value: 35 Find all pairs in the said list whose sum is equal to a target value: [{20, 15}, {16, 19}, {17, 18}, {17, 18}, {16, 19}, {20, 15}] Original list of numbers: [1, 2, 3, 4, 5] Target value: 5 Find all pairs in the said list whose sum is equal to a target value: [{1, 4}, {2, 3}, {2, 3}, {1, 4}]
Flowchart:
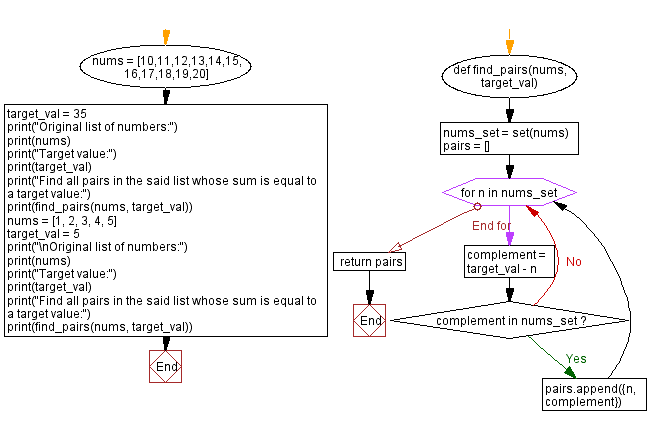
For more Practice: Solve these Related Problems:
- Write a Python program to find and print all unique pairs from a list that add up to a specified target sum.
- Write a Python program to use a two-pointer technique on a sorted list to identify pairs whose sum equals a given value.
- Write a Python program to implement a function that returns a list of tuple pairs that sum to the target value, ensuring no duplicates.
- Write a Python program to use dictionary mapping to find pairs that add up to a target sum in a single pass through the list.
Go to:
Previous: Unique words and frequency from a given list of strings.
Next: Longest common prefix of all the strings.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.