Python: Maximum product of two numbers among all pairs in a list
Write a Python program to find the two numbers whose product is maximum among all the pairs in a given list of numbers. Use the Python set.
Sample Solution:
Python Code:
# Define a function 'max_product' that takes a list of integers 'nums' as input.
def max_product(nums):
# Create a set 'nums_set' to efficiently check for unique numbers in the input list.
nums_set = set(nums)
# Initialize 'max_product' to negative infinity and 'max_num1' and 'max_num2' to None.
max_product = float('-inf')
max_num1 = None
max_num2 = None
# Iterate over the unique numbers in 'nums_set'.
for n1 in nums_set:
for n2 in nums_set:
# Check if 'n1' and 'n2' are distinct and their product is greater than 'max_product'.
if n1 != n2 and n1 * n2 > max_product:
# Update 'max_product' with the new maximum product value.
max_product = n1 * n2
# Store the numbers that produced the maximum product in 'max_num1' and 'max_num2'.
max_num1 = n1
max_num2 = n2
# Return a tuple containing the two numbers that produced the maximum product.
return (max_num1, max_num2)
# Define a list of integers 'nums' for testing.
nums = [1, 2, 3, 4, 5, 6, 7, 8, 9]
print("Original list of integers:")
print(nums)
# Call the 'max_product' function and print the result for the list of integers.
print("Maximum product of two numbers among all pairs in the said list:")
print(max_product(nums))
# Repeat the process for a different set of integers.
nums = [1, -2, -3, 4, 5, -6, 7, -8, 9]
print("\nOriginal list of integers:")
print(nums)
print("Maximum product of two numbers among all pairs in the said list:")
print(max_product(nums))
Sample Output:
Original list of integers: [1, 2, 3, 4, 5, 6, 7, 8, 9] Maximum product of two numbers among all pairs in the said list: (8, 9) Original list of integers: [1, -2, -3, 4, 5, -6, 7, -8, 9] Maximum product of two numbers among all pairs in the said list: (7, 9)
Flowchart:
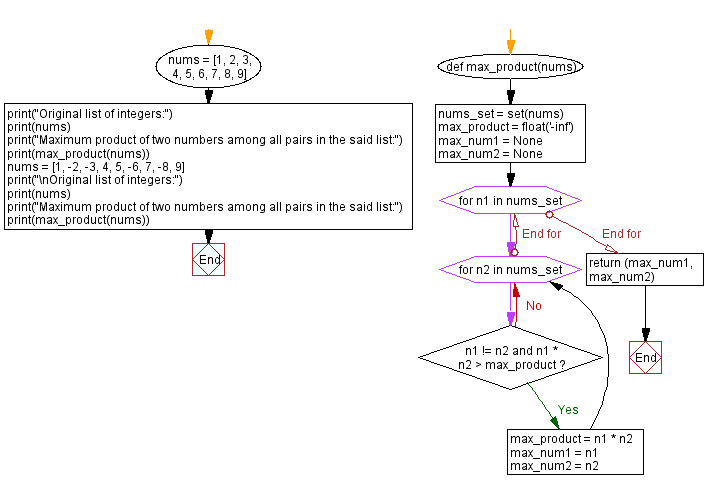
Python Code Editor:
Previous: Longest common prefix of all the strings.
Next: Find the missing numbers between the two sets.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics