Python: Remove items from Python set
4. Remove Item(s) from a Given Set
Write a Python program to remove item(s) from a given set.
Visual Presentation:
Sample Solution-1:
Python Code:
Remove single element from a given set:
# Create a set 'num_set' with the elements 0, 1, 3, 4, and 5 using a list:
num_set = set([0, 1, 3, 4, 5])
# Print a message to indicate the original set:
print("Original set:")
# Print the contents of the 'num_set':
print(num_set)
# Remove and return the first element from 'num_set' using the 'pop' method:
num_set.pop()
# Print a message to indicate the set after removing the first element:
print("\nAfter removing the first element from the said set:")
# Print the updated 'num_set' without the first element:
print(num_set)
Sample Output:
Original set: {0, 1, 3, 4, 5} After removing the first element from the said set: {1, 3, 4, 5}
Sample Solution-2:
Python Code:
Remove all elements from a given set:
# Define a function 'remove_all_elements' that takes a set 'num_set' as an argument:
def remove_all_elements(num_set):
# Use a 'while' loop to remove elements from 'num_set' as long as it is not empty:
while num_set:
num_set.pop()
# Return the empty 'num_set' after removing all elements:
return num_set
# Create a set 'num_set' with the elements 0, 1, 3, 4, and 5 using a list:
num_set = set([0, 1, 3, 4, 5])
# Print a message to indicate the original set:
print("Original set:")
# Print the contents of the 'num_set':
print(num_set)
# Print a message to indicate the set after removing all elements:
print("\nAfter removing all elements from the said set:")
# Call the 'remove_all_elements' function to remove all elements from 'num_set' and print the resulting set (should be empty):
print(remove_all_elements(num_set))
Sample Output:
Original set: {0, 1, 3, 4, 5} After removing all elements from the said set: set()
Flowchart:
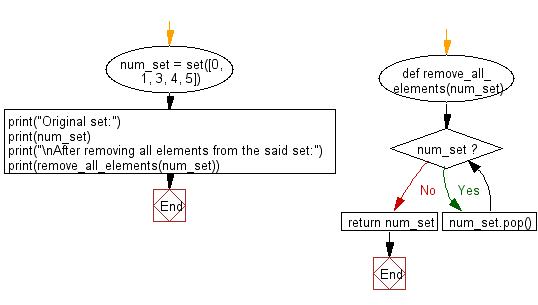
For more Practice: Solve these Related Problems:
- Write a Python program to remove a list of specified elements from a set using the difference_update() method.
- Write a Python program to delete items from a set that are less than a given threshold.
- Write a Python program to iterate over a set and remove any element that meets a particular condition using set comprehension.
- Write a Python program to remove elements from a set using a loop and the discard() method, then print the resulting set.
Go to:
Previous: Write a Python program to add member(s) in a set.
Next: Write a Python program to remove an item from a set if it is present in the set.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.