Python: Intersection of sets
6. Create an Intersection of Sets
Write a Python program to create an intersection of sets.
In mathematics, the intersection of two sets A and B, denoted by A ∩ B, is the set containing all elements of A that also belong to B (or equivalently, all elements of B that also belong to A).
In set-builder notation, A ∩ B = {x ∈ U : x ∈ A and x ∈ B}.
The Venn diagram for A ∩ B is shown to the right where the shaded region represents the set A ∩ B.
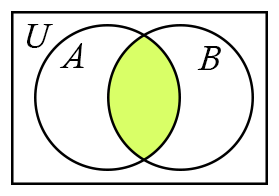
Example: Let A = {a, b, c, d} and B = {b, d, e}. Then A ∩ B = {b, d}. The elements b and d are the only elements that are in both sets A and B.
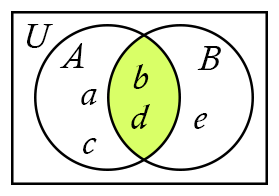
Visual Presentation:
Sample Solution-1:
Python Code:
Sample Output:
Original set elements: {'green', 'blue'} {'blue', 'yellow'} Intersection of two said sets: {'blue'}
Sample Solution-2:
Python Code:
Sample Output:
Original set elements: {'green', 'blue'} {'yellow', 'blue'} Intersection of two said sets: ['blue']
Flowchart:
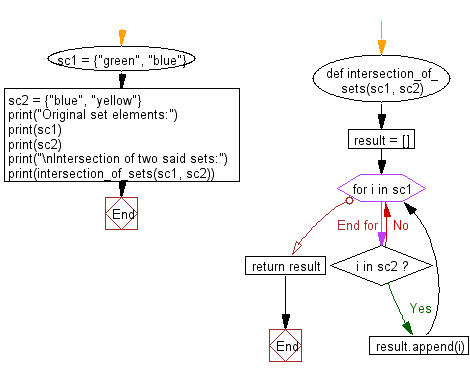
Sample Solution-3:
Python Code:
Sample Output:
Original set elements: {'green', 'blue'} {'yellow', 'blue'} Intersection of two said sets: ['blue']
For more Practice: Solve these Related Problems:
- Write a Python program to compute the intersection of two sets of integers using the & operator.
- Write a Python program to find the common elements between two sets using the intersection() method.
- Write a Python program to implement a function that returns the intersection of multiple sets provided as arguments.
- Write a Python program to use list comprehension and set conversion to compute the intersection of two lists.
Go to:
Previous: Write a Python program to remove an item from a set if it is present in the set.
Next: Write a Python program to create a union of sets.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.