Python - Create SQLAlchemy model 'Student' with fields
1. Student Model Creation
Write a Python program to create a SQLAlchemy model 'Student' with fields: 'id', 'studentname', and 'email'.
Sample Solution:
Code:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Create a SQLite in-memory database
engine = create_engine('sqlite:///testdatabase')
# Create a base class for declarative models
Base = declarative_base()
# Define the Student model
class Student(Base):
__tablename__ = 'students'
id = Column(Integer, primary_key=True, autoincrement=True)
studentname = Column(String, nullable=False)
email = Column(String, nullable=False)
# Create the table in the database
Base.metadata.create_all(engine)
# Create a session to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
# Add a new Student to the database
new_student = Student(id=15,studentname='Zhv Twibfnc', email='zhv@w3resource.com')
session.add(new_student)
session.commit()
# Query and print all Students from the database
result = session.query(Student).all()
for Student in result:
print(Student.id, Student.studentname, Student.email)
# Close the session
session.close()
Output:
15 Zhv Twibfnc zhv@w3resource.com
The above exercise shows how to use SQLAlchemy's ORM, models, sessions and querying to interact with a database without writing raw SQL, in a Pythonic way.
Flowchart:
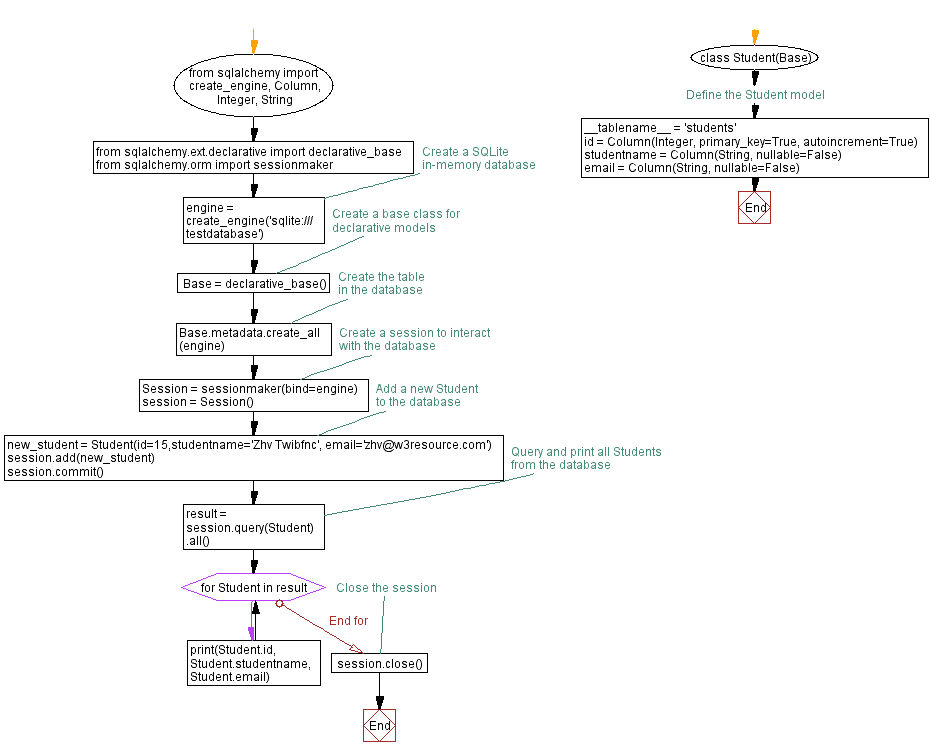
For more Practice: Solve these Related Problems:
- Write a Python program to define a SQLAlchemy model 'Student' with fields 'id' (primary key), 'studentname', and 'email', and then print the generated SQL CREATE TABLE statement.
- Write a Python function that creates a 'Student' model with a unique constraint on the 'email' field, and then outputs the table's metadata.
- Write a Python script to create a 'Student' model in an in-memory SQLite database and list all tables to verify that the 'Student' table has been created.
- Write a Python program that defines a 'Student' model with custom data types for each field, then prints the SQLAlchemy table columns and their properties.
Python Code Editor :
Previous: Python SQLAlchemy Exercises Home.
Next: Python Program: Add new records to database table.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.