Python program to update a table field
Write a Python program that updates a student's email in the 'students' table based on their id.
Sample Solution:
Code:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
# Create a SQLite database named students.db
engine = create_engine('sqlite:///testdatabase.db', echo=False)
# Create a base class for declarative models
Base = declarative_base()
# Define the Student model
class Student(Base):
__tablename__ = 'students'
id = Column(Integer, primary_key=True)
studentname = Column(String, nullable=False)
email = Column(String, nullable=False)
# Create a session to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
def update_student_email(student_id, new_email):
student = session.query(Student).filter_by(id=student_id).first()
if student:
student.email = new_email
session.commit()
print("Student email updated successfully")
else:
print("Student with ID {student_id} not found")
# Update a user's email based on their ID
student_id = 22 # Replace with the desired student ID
new_email = 'hughie_glauco@example.com' # Replace with the new email
update_student_email(student_id, new_email)
# Close the session
session.close()
Output:
Student email updated successfully
Flowchart:
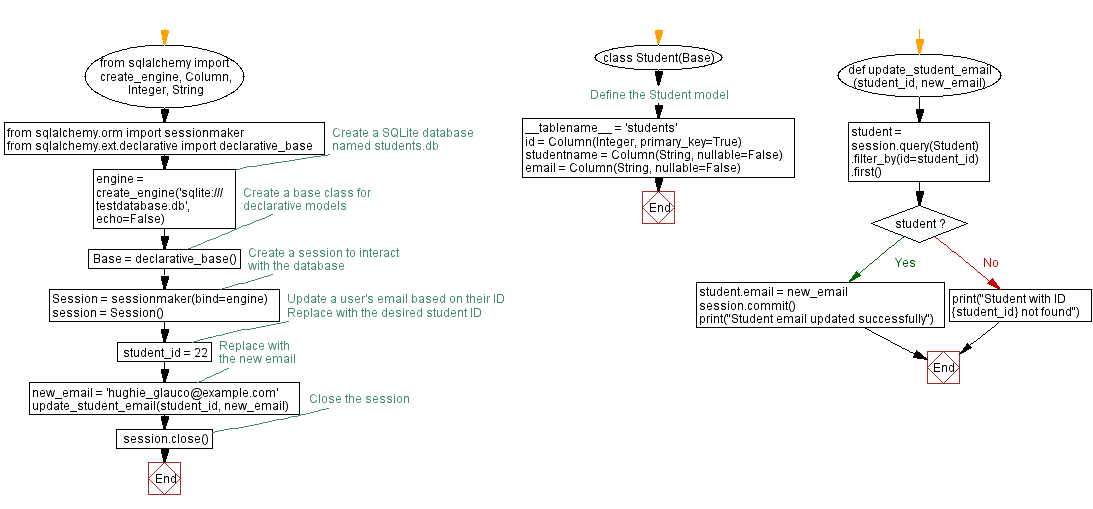
Previous: Python Program: Add new records to database table.
Next: Python program to delete a record from a table.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics