Python program: Retrieve items with price greater than
Python SQLAlchemy: Exercise-7 with Solution
Write a Python program to retrieve items with a price greater than a certain value from the 'items' table using the SQLAlchemy model.
Sample Solution:
Code:
from sqlalchemy import create_engine, Column, Integer, Float, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
# Create a SQLite database named shop.db
engine = create_engine('sqlite:///shop2.db', echo=False)
# Create a base class for declarative models
Base = declarative_base()
# Define the Item model
class Item(Base):
__tablename__ = 'items'
item_id = Column(Integer, primary_key=True)
item_name = Column(String, nullable=False)
item_price = Column(Float, nullable=False)
item_quantity = Column(Integer, nullable=False)
# Create a session to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
def items_with_price_above(threshold_price):
items = session.query(Item).filter(Item.item_price > threshold_price).all()
return items
# Retrieve items with a price greater than a certain value
threshold_price = 2.0 # Replace with the desired threshold price
items_above_threshold = items_with_price_above(threshold_price)
# Print the retrieved items
print(f"Items with a price greater than {threshold_price}:")
for item in items_above_threshold:
print(f"Item ID: {item.item_id}, Name: {item.item_name}, Price: {item.item_price}")
# Close the session
session.close()
Output:
Items with a price greater than 2.0: Item ID: 1, Name: Pizza, Price: 15.82 Item ID: 2, Name: Burger, Price: 2.82
Flowchart:
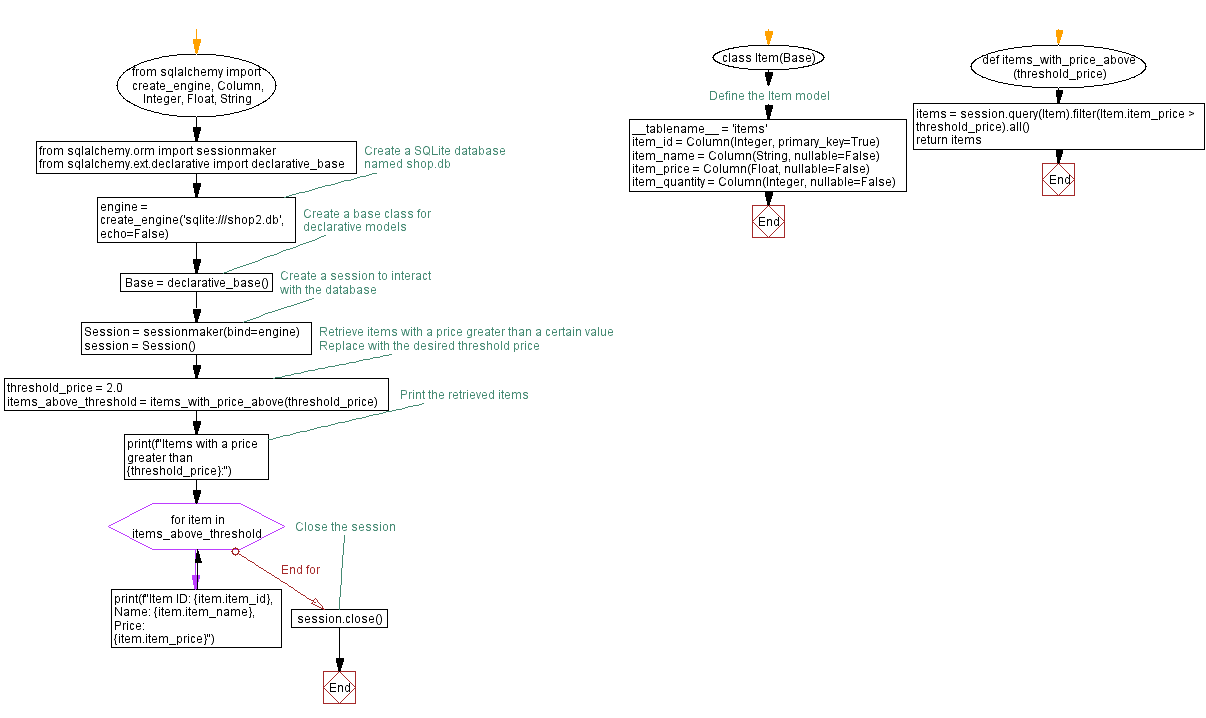
Previous: Create SQLAlchemy models and populating tables.
Next: Update item quantity using SQLAlchemy.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/sqlalchemy/python-sqlalchemy-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics