Python: Change a given string to a new string where the first and last chars have been exchanged
Swap first and last chars of a string.
Write a Python program to change a given string to a newly string where the first and last chars have been exchanged.
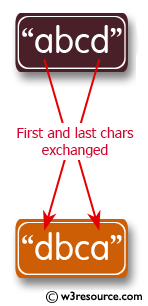
Sample Solution:
Python Code:
# Define a function named change_string that takes one argument, 'str1'.
def change_string(str1):
# Return a modified version of the input string 'str1' by rearranging its characters.
# It takes the last character and moves it to the front, while keeping the middle characters unchanged.
return str1[-1:] + str1[1:-1] + str1[:1]
# Call the change_string function with different input strings and print the results.
print(change_string('abcd')) # Output: 'dbca'
print(change_string('12345')) # Output: '52341'
Sample Output:
dbca 52341
Flowchart:
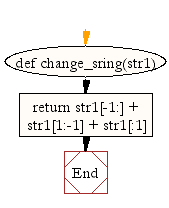
Python Code Editor:
Previous: Write a Python program to remove the nth index character from a nonempty string.
Next: Write a Python program to remove the characters which have odd index values of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics