Python: Check whether any word in a given sting contains duplicate characrters or not
Python String: Exercise-100 with Solution
Write a Python program to check whether any word in a given string contains duplicate characters or not. Return True or False.
Sample Solution-1:
Python Code:
# Define a function to check if any word in a string contains duplicate characters
def duplicate_letters(text):
# Split the input string into a list of words
word_list = text.split()
# Iterate through each word in the list
for word in word_list:
# Check if the length of the word is greater than the number of unique characters in the word
if len(word) > len(set(word)):
# If true, the word contains duplicate characters, return False
return False
# If no word with duplicate characters is found, return True
return True
# Initialize a string
text = "Filter out the factorials of the said list."
# Print the original text
print("Original text:")
print(text)
# Print a message indicating the check for duplicate characters in any word
print("Check whether any word in the said sting contains duplicate characters or not!")
# Call the function to check for duplicate characters and print the result
print(duplicate_letters(text))
# Repeat the process with different input strings
text = "Python Exercise."
print("\nOriginal text:")
print(text)
print("Check whether any word in the said sting contains duplicate characters or not!")
print(duplicate_letters(text))
text = "The wait is over."
print("\nOriginal text:")
print(text)
print("Check whether any word in the said sting contains duplicate characters or not!")
print(duplicate_letters(text))
Sample Output:
Original text: Filter out the factorials of the said list. Check whether any word in the said sting contains duplicate characrters or not! False Original text: Python Exercise. Check whether any word in the said sting contains duplicate characrters or not! False Original text: The wait is over. Check whether any word in the said sting contains duplicate characrters or not! True
Flowchart:
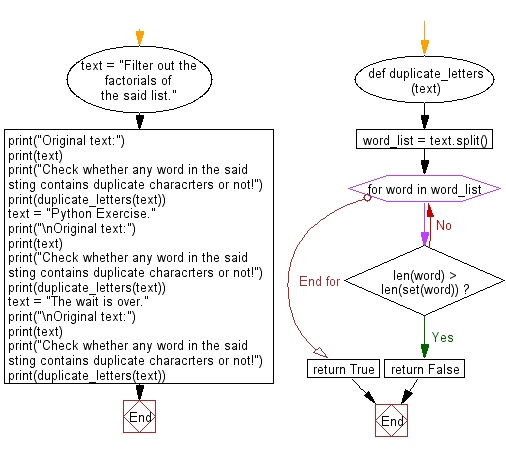
Sample Solution-2:
Python Code:
# Define a concise function to check if any word in a string contains duplicate characters
def duplicate_letters(text):
# Use a generator expression and the 'all()' function to check if all words meet the condition
return all(len(word) == len(set(word)) for word in text.split())
# Initialize a string
text = "Filter out the factorials of the said list."
# Print the original text
print("Original text:")
print(text)
# Print a message indicating the check for duplicate characters in any word
print("Check whether any word in the said sting contains duplicate characters or not!")
# Call the function to check for duplicate characters and print the result
print(duplicate_letters(text))
# Repeat the process with different input strings
text = "Python Exercise."
print("\nOriginal text:")
print(text)
print("Check whether any word in the said sting contains duplicate characters or not!")
print(duplicate_letters(text))
text = "The wait is over."
print("\nOriginal text:")
print(text)
print("Check whether any word in the said sting contains duplicate characters or not!")
print(duplicate_letters(text))
Sample Output:
Original text: Filter out the factorials of the said list. Check whether any word in the said sting contains duplicate characrters or not! False Original text: Python Exercise. Check whether any word in the said sting contains duplicate characrters or not! False Original text: The wait is over. Check whether any word in the said sting contains duplicate characrters or not! True
Flowchart:
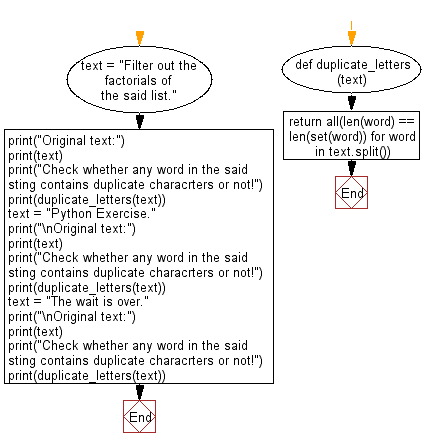
Python Code Editor:
Previous: Write a Python program to split a given multiline string into a list of lines.
Next: Write a Python program to add two strings as they are numbers (Positive integer values). Return a message if the numbers are string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-100.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics