Python Exercise: Add two strings as they are numbers (Positive integer values)
Add two strings as numbers.
Write a Python program to add two strings as if they were numbers (positive integer values). Return a message if the numbers are strings.
Sample Solution-1:
Python Code:
# Define a function to add two numbers represented as strings
def test(n1, n2):
# Add '0' to the beginning of each input string to handle negative numbers
n1, n2 = '0' + n1, '0' + n2
# Check if both modified strings are numeric
if (n1.isnumeric() and n2.isnumeric()):
# If true, convert the strings to integers, add them, and return the result as a string
return str(int(n1) + int(n2))
else:
# If not numeric, return an error message
return 'Error in input!'
# Test the function with different input strings and print the results
print(test("10", "32"))
print(test("10", "22.6"))
print(test("100", "-200"))
Sample Output:
42 Error in input! Error in input!
Flowchart:
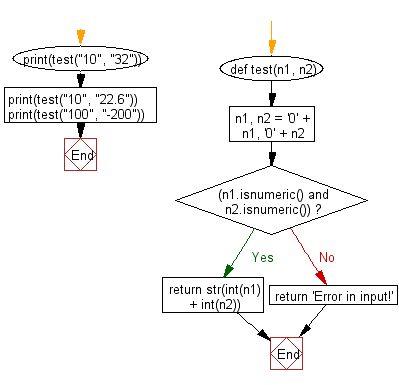
Sample Solution-2:
Python Code:
# Define a function to add two numbers represented as strings
def test(n1, n2):
# Check if n1 is an empty string, replace it with '0' if true
if n1 == '':
n1 = '0'
# Check if n2 is an empty string, replace it with '0' if true
if n2 == '':
n2 = '0'
# Check if both modified strings are numeric
if n1.isdigit() and n2.isdigit():
# If true, convert the strings to integers, add them, and return the result as a string
return str(int(n1) + int(n2))
else:
# If not numeric, return an error message
return 'Error in input!'
# Test the function with different input strings and print the results
print(test("23", "43"))
print(test("12", "11.6"))
print(test("200", "-100"))
Sample Output:
66 Error in input! Error in input!
Flowchart:
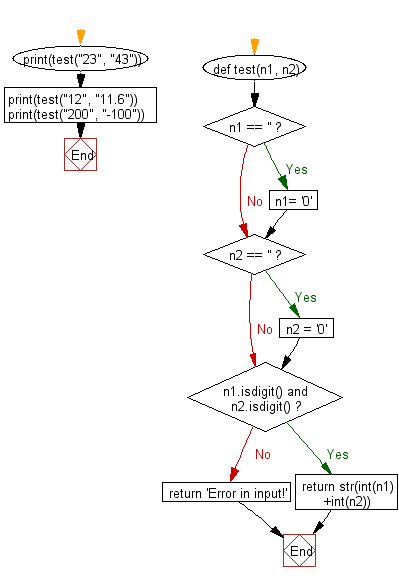
For more Practice: Solve these Related Problems:
- Write a Python program to add two numbers provided as strings, returning their sum as a string.
- Write a Python program to validate input strings to ensure they represent positive integers before summing them.
- Write a Python program to implement string addition without converting the inputs to integers using iterative digit processing.
- Write a Python program to handle edge cases where one or both input strings are empty and output an error message.
Go to:
Previous Python Exercise: Check whether any word in a given sting contains duplicate characrters or not.
Next Python Exercise: Remove punctuations from a string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.