Python Exercises: Replace a word with hash characters in a string
Replace long words with hash.
Write a Python program to replace each character of a word of length five and more with a hash character (#).
Sample Solution-1:
Python Code:
# Define a function to replace words with hash characters if their length is five or more
def test(text):
# Iterate through each word in the string
for i in text.split():
# Check if the length of the word is greater than or equal to 5
if len(i) >= 5:
# If true, replace the word with '#' repeated for the length of the word
text = text.replace(i, "#" * len(i))
# Return the modified string
return text
# Initialize a string with words of varying lengths
text = "Count the lowercase letters in the said list of words:"
print("Original string:", text)
# Print a message indicating the replacement of words with hash characters
print("Replace words (length five or more) with hash characters in the said string:")
# Call the function to replace words and print the result
print(test(text))
# Repeat the process with a different string
text = "Python - Remove punctuations from a string:"
print("\nOriginal string:", text)
# Print a message indicating the replacement of words with hash characters
print("Replace words (length five or more) with hash characters in the said string:")
# Call the function to replace words and print the result
print(test(text))
Sample Output:
Original string: Count the lowercase letters in the said list of words: Replace words (length five or more) with hash characters in the said string: ##### the ######### ####### in the said list of ###### Original string: Python - Remove punctuations from a string: Replace words (length five or more) with hash characters in the said string: ###### - ###### ############ from a #######
Flowchart:
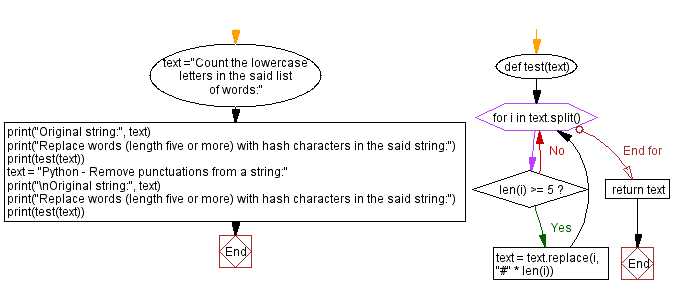
Sample Solution-2:
Python Code:
# Define a concise function to replace words with hash characters if their length is five or more
def test(text):
# Use a generator expression to replace words based on their length
return ' '.join('#' * len(i) if len(i) >= 5 else i for i in text.split(' '))
# Initialize a string with words of varying lengths
text ="Count the lowercase letters in the said list of words:"
print("Original string:", text)
# Print a message indicating the replacement of words with hash characters
print("Replace words (length five or more) with hash characters in the said string:")
# Call the function to replace words and print the result
print(test(text))
# Repeat the process with a different string
text = "Python - Remove punctuations from a string:"
print("\nOriginal string:", text)
# Print a message indicating the replacement of words with hash characters
print("Replace words (length five or more) with hash characters in the said string:")
# Call the function to replace words and print the result
print(test(text))
Sample Output:
Original string: Count the lowercase letters in the said list of words: Replace words (length five or more) with hash characters in the said string: ##### the ######### ####### in the said list of ###### Original string: Python - Remove punctuations from a string: Replace words (length five or more) with hash characters in the said string: ###### - ###### ############ from a #######
Flowchart:
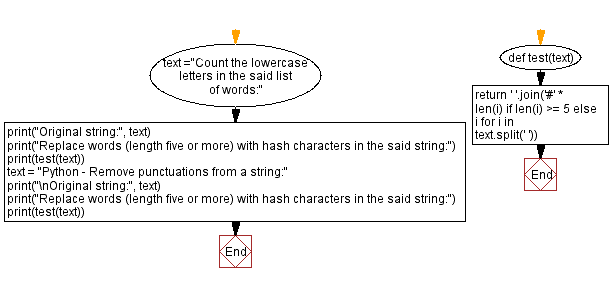
Python Code Editor:
Previous Python Exercise: Remove punctuations from a string.
Next Python Exercise: Capitalize the first letter and lowercases the rest.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics